springboot stripe
时间: 2024-03-03 18:45:58 浏览: 19
Spring Boot是一个用于构建Java应用程序的开源框架,它简化了Java应用程序的开发过程。Stripe是一家提供在线支付解决方案的公司,它提供了一套API和工具,帮助开发者轻松地集成支付功能到他们的应用程序中。
在Spring Boot中使用Stripe可以通过Stripe的Java客户端库来实现。你可以在你的项目中添加Stripe的依赖,然后使用Stripe提供的API来进行支付操作。以下是使用Spring Boot和Stripe集成的一般步骤:
1. 添加Stripe依赖:在你的Spring Boot项目的pom.xml文件中添加Stripe的依赖。
2. 配置Stripe API密钥:在你的应用程序的配置文件中配置Stripe的API密钥,这个密钥可以在Stripe的开发者控制台中获取。
3. 创建支付请求:使用Stripe提供的API创建支付请求,包括支付金额、货币类型、付款方式等信息。
4. 处理支付结果:根据Stripe返回的支付结果,处理支付成功或失败的逻辑。
5. 安全性考虑:在处理支付过程中,确保数据传输和存储的安全性,例如使用HTTPS协议进行数据传输,加密敏感信息等。
相关问题
springboot连携Stripe
Spring Boot是一个用于构建Java应用程序的开源框架,它简化了Java应用程序的开发过程。Stripe是一家提供在线支付解决方案的公司,它提供了一套API和工具,帮助开发者轻松地集成支付功能到他们的应用程序中。
要在Spring Boot应用程序中集成Stripe,可以按照以下步骤进行操作:
1. 添加Stripe依赖:在项目的pom.xml文件中添加Stripe的依赖项。可以通过在<dependencies>标签内添加以下代码来实现:
```xml
<dependency>
<groupId>com.stripe</groupId>
<artifactId>stripe-java</artifactId>
<version>20.60.0</version>
</dependency>
```
2. 配置Stripe API密钥:在应用程序的配置文件(如application.properties或application.yml)中添加Stripe的API密钥。可以在Stripe的开发者控制台中获取API密钥。
```properties
stripe.apiKey=YOUR_STRIPE_API_KEY
```
3. 创建Stripe服务:创建一个StripeService类,用于处理与Stripe API的交互。可以使用Stripe的Java库来执行各种操作,如创建支付Intent、创建订阅等。
```java
@Service
public class StripeService {
@Value("${stripe.apiKey}")
private String apiKey;
public PaymentIntent createPaymentIntent(int amount, String currency) throws StripeException {
Stripe.apiKey = apiKey;
PaymentIntentCreateParams params = new PaymentIntentCreateParams.Builder()
.setAmount(amount)
.setCurrency(currency)
.build();
return PaymentIntent.create(params);
}
// 其他Stripe相关操作的方法...
}
```
4. 使用Stripe服务:在需要使用Stripe支付的地方,注入StripeService并调用相应的方法。
```java
@RestController
public class PaymentController {
@Autowired
private StripeService stripeService;
@PostMapping("/payment")
public PaymentIntent createPayment(@RequestParam int amount, @RequestParam String currency) throws StripeException {
return stripeService.createPaymentIntent(amount, currency);
}
// 其他支付相关的接口...
}
```
这样,你就可以在Spring Boot应用程序中使用Stripe进行支付了。当然,以上只是一个简单的示例,实际使用中可能还需要处理更多的逻辑和错误情况。
golang stripe
Golang Stripe是一个用于处理支付的开源库,它提供了与Stripe支付平台进行交互的功能。Stripe是一家全球性的在线支付解决方案提供商,它允许开发者轻松地集成支付功能到他们的应用程序中。
Golang Stripe库提供了一系列API方法,可以用于创建和管理Stripe对象,如顾客、支付方式、订阅和支付等。使用该库,开发者可以方便地进行支付操作,包括创建支付订单、处理支付回调、查询支付状态等。
Golang Stripe库的特点包括:
1. 简单易用:提供了简洁的API方法,使得开发者能够轻松地与Stripe进行交互。
2. 完善的文档:Stripe官方提供了详细的文档和示例代码,帮助开发者快速上手和集成。
3. 安全可靠:Stripe采用了多种安全措施来保护用户的支付信息,包括数据加密、PCI DSS合规等。
使用Golang Stripe库,你可以实现以下功能:
1. 创建和管理顾客信息:可以创建新的顾客、更新顾客信息、查询顾客信息等。
2. 管理支付方式:可以添加、删除和查询顾客的支付方式,如信用卡、银行账户等。
3. 处理支付订单:可以创建支付订单、查询订单状态、退款等。
4. 管理订阅:可以创建和管理订阅计划,实现定期支付功能。
相关推荐
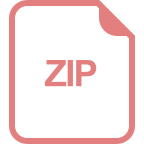
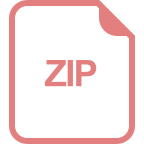
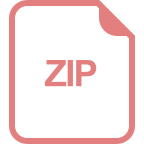












