用C++写一个让用户输入数字,并将数字放在链表里的代码
时间: 2024-09-09 14:14:53 浏览: 87
在C++中,创建一个链表并允许用户输入数字,然后将这些数字添加到链表中,可以通过定义一个简单的链表结构体和一些操作函数来实现。以下是一个示例代码:
```cpp
#include <iostream>
// 定义链表节点结构体
struct ListNode {
int value;
ListNode *next;
ListNode(int x) : value(x), next(nullptr) {} // 构造函数
};
// 定义链表类
class LinkedList {
private:
ListNode *head; // 链表头指针
public:
LinkedList() : head(nullptr) {} // 构造函数
// 向链表末尾添加元素
void append(int value) {
if (!head) {
head = new ListNode(value);
return;
}
ListNode *current = head;
while (current->next) {
current = current->next;
}
current->next = new ListNode(value);
}
// 打印链表元素
void print() {
ListNode *current = head;
while (current) {
std::cout << current->value << " -> ";
current = current->next;
}
std::cout << "nullptr" << std::endl;
}
// 析构函数,用于释放链表内存
~LinkedList() {
ListNode *current = head;
while (current) {
ListNode *toDelete = current;
current = current->next;
delete toDelete;
}
}
};
int main() {
LinkedList list;
int num;
std::cout << "请输入数字,输入非数字结束:" << std::endl;
while (std::cin >> num) {
list.append(num);
}
// 清理输入流状态
std::cin.clear();
// 忽略剩余输入直到行尾
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n');
std::cout << "链表中的数字如下:" << std::endl;
list.print();
return 0;
}
```
这段代码首先定义了一个链表节点结构体`ListNode`,每个节点包含一个整数类型的数据成员`value`和一个指向下一个节点的指针`next`。`LinkedList`类封装了链表操作,包括添加元素到链表末尾的`append`方法和打印链表的`print`方法。`main`函数负责接收用户输入并创建链表。
用户可以连续输入数字,每输入一个数字就会被添加到链表的末尾。当用户输入非数字字符时,程序会停止接收输入,并打印出链表中的所有数字。
阅读全文
相关推荐


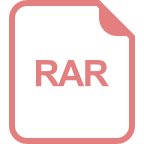













