能否提供一个用VC++实现的简单凯撒加密算法的示例代码?
时间: 2024-10-25 10:18:49 浏览: 18
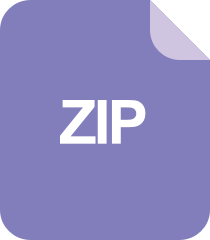
凯撒加密算法的C语言实现.zip
当然可以,这里是一个简单的VC++(Visual C++)实现的凯撒加密算法示例代码。这个例子将对给定的字符串每个字符进行位移(Shift)操作:
```cpp
#include <iostream>
#include <string>
// 函数声明:用于加密字符串
std::string caesarEncrypt(const std::string& plaintext, int shift);
int main() {
std::string input = "Hello, World!";
int key = 3; // 加密密钥,比如3表示向右移动三个位置
std::string encrypted = caesarEncrypt(input, key);
std::cout << "Original Text: " << input << "\n";
std::cout << "Encrypted Text: " << encrypted << "\n";
return 0;
}
// 实现凯撒加密函数
std::string caesarEncrypt(const std::string& plaintext, int shift) {
std::string result = "";
for (char c : plaintext) {
if ('A' <= c && c <= 'Z') { // 大写字母处理
result += static_cast<char>((c - 'A' + shift) % 26 + 'A');
} else if ('a' <= c && c <= 'z') { // 小写字母处理
result += static_cast<char>((c - 'a' + shift) % 26 + 'a');
} else { // 非字母字符保持原样
result += c;
}
}
return result;
}
```
在这个代码中,`caesarEncrypt`函数接受一个明文字符串和一个密钥,然后通过将大写字母和小写字母分别循环移动指定的位数来加密。非字母字符会保持不变。
阅读全文
相关推荐
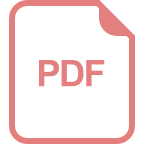
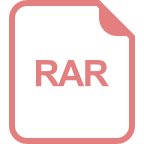















