我要开发一个工具脚本,每个月初需要下载这个网站上展示的数据,用java开发的话,需要怎么做?能从搭建框架到代码编写 说说具体步骤么?
时间: 2024-11-30 08:16:59 浏览: 2
要开发一个Java工具脚本来每月定时下载中国国际贸易单一窗口网站上的数据,你可以按照以下步骤进行:
### 1. 搭建开发环境
- **安装Java开发工具**:确保已经安装了JDK和IDE(如IntelliJ IDEA或Eclipse)。
- **创建项目**:在IDE中创建一个新的Java项目。
### 2. 添加依赖
- **Maven或Gradle**:如果你使用Maven或Gradle作为构建工具,可以在`pom.xml`或`build.gradle`文件中添加必要的依赖项。例如,用于HTTP请求的Apache HttpClient库:
```xml
<!-- Maven -->
<dependency>
<groupId>org.apache.httpcomponents</groupId>
<artifactId>httpclient</artifactId>
<version>4.5.13</version>
</dependency>
```
或者:
```groovy
// Gradle
implementation 'org.apache.httpcomponents:httpclient:4.5.13'
```
### 3. 编写HTTP请求代码
- **导入必要的包**:
```java
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
import java.io.FileOutputStream;
import java.io.InputStream;
import java.io.IOException;
```
- **编写下载方法**:
```java
public class DataDownloader {
private static final String URL = "https://www.singlewindow.cn/#/parameterDetail?pqcode=CusComplex";
public void downloadData() throws IOException {
try (CloseableHttpClient httpClient = HttpClients.createDefault()) {
HttpGet request = new HttpGet(URL);
try (CloseableHttpResponse response = httpClient.execute(request)) {
if (response.getStatusLine().getStatusCode() == 200) {
InputStream inputStream = response.getEntity().getContent();
FileOutputStream outputStream = new FileOutputStream("data.html");
byte[] buffer = new byte[1024];
int length;
while ((length = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, length);
}
outputStream.close();
System.out.println("Data downloaded successfully.");
} else {
System.out.println("Failed to download data. Status code: " + response.getStatusLine().getStatusCode());
}
}
}
}
}
```
### 4. 定时任务调度
- **使用Java Timer类**:
```java
import java.util.Timer;
import java.util.TimerTask;
public class Main {
public static void main(String[] args) {
Timer timer = new Timer();
TimerTask task = new TimerTask() {
@Override
public void run() {
DataDownloader downloader = new DataDownloader();
try {
downloader.downloadData();
} catch (IOException e) {
e.printStackTrace();
}
}
};
// 设置定时任务,每月1号凌晨0点执行
long initialDelay = calculateInitialDelay();
long period = 2678400000L; // 一个月的时间(毫秒)
timer.schedule(task, initialDelay, period);
}
private static long calculateInitialDelay() {
// 计算下一次1号0点的时间与当前时间的差值
Calendar calendar = Calendar.getInstance();
calendar.set(Calendar.DAY_OF_MONTH, 1);
calendar.set(Calendar.HOUR_OF_DAY, 0);
calendar.set(Calendar.MINUTE, 0);
calendar.set(Calendar.SECOND, 0);
calendar.set(Calendar.MILLISECOND, 0);
if (calendar.getTimeInMillis() <= System.currentTimeMillis()) {
calendar.add(Calendar.MONTH, 1);
}
return calendar.getTimeInMillis() - System.currentTimeMillis();
}
}
```
### 5. 测试和调试
- **运行程序**:在IDE中运行主类`Main`,确保数据能够成功下载并保存到指定路径。
- **日志记录**:可以添加日志记录来帮助调试和监控程序运行情况。
### 6. 部署和维护
- **部署到服务器**:将编译好的jar文件部署到生产服务器,并确保定时任务正常运行。
- **监控和维护**:定期检查程序运行日志,确保没有异常情况发生。
通过以上步骤,你可以实现一个每月定时下载中国国际贸易单一窗口网站数据的Java工具脚本。希望这些信息对你有所帮助!如果有任何问题,请随时联系我。
阅读全文
相关推荐
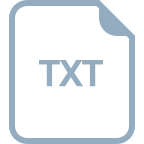
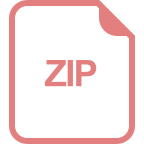
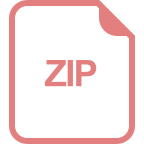
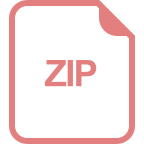
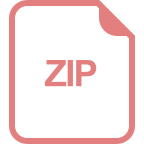
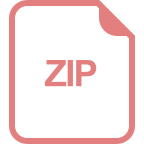
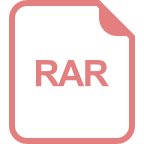
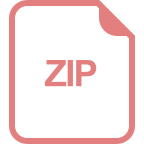
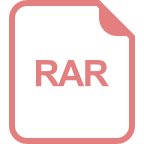
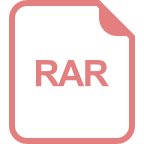
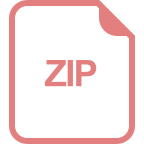
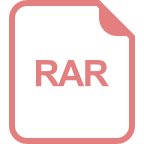
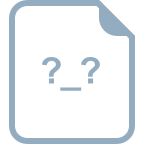
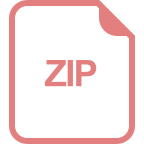
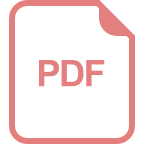
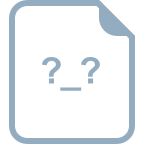
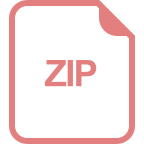