vb.net UDP多线程发送
时间: 2023-07-31 15:05:13 浏览: 130
在 VB.NET 中,你可以使用 System.Net.Sockets 命名空间来实现 UDP 多线程发送。下面是一个简单的示例代码:
```vb
Imports System.Net
Imports System.Net.Sockets
Imports System.Threading
Public Class UDPSender
Private Shared sendDone As New ManualResetEvent(False)
Public Shared Sub Main()
Dim remoteEP As New IPEndPoint(IPAddress.Parse("目标IP地址"), 目标端口号)
Dim client As New UdpClient()
' 启动多个线程同时发送 UDP 数据包
For i As Integer = 0 To 4
Dim thread As New Thread(AddressOf Send)
thread.Start(New SendState(client, remoteEP, "消息" & i.ToString()))
Next
sendDone.WaitOne() ' 等待所有线程完成发送
client.Close()
End Sub
Private Shared Sub Send(state As Object)
Dim sendState As SendState = DirectCast(state, SendState)
Dim client As UdpClient = sendState.Client
Dim remoteEP As IPEndPoint = sendState.RemoteEP
Dim message As String = sendState.Message
Dim bytes As Byte() = Encoding.ASCII.GetBytes(message)
client.Send(bytes, bytes.Length, remoteEP)
Console.WriteLine("Sent: {0}", message)
sendDone.Set() ' 通知主线程发送完成
End Sub
End Class
Public Class SendState
Public Property Client As UdpClient
Public Property RemoteEP As IPEndPoint
Public Property Message As String
Public Sub New(client As UdpClient, remoteEP As IPEndPoint, message As String)
Me.Client = client
Me.RemoteEP = remoteEP
Me.Message = message
End Sub
End Class
```
在上面的示例中,我们创建了一个名为 "UDPSender" 的类,并在 Main 方法中实现了多线程发送 UDP 数据包的逻辑。你需要将 "目标IP地址" 和 "目标端口号" 分别替换为你要发送到的目标地址和端口号。
每个线程通过创建一个名为 "Send" 的方法来发送 UDP 数据包。我们使用 ManualResetEvent 来等待所有线程完成发送操作。在发送完成后,通过调用 sendDone.Set() 来通知主线程。
请注意,这只是一个基本示例,你可以根据自己的需求进行修改和扩展。同时,为了保证线程安全性,你可能需要在发送数据包之前添加适当的同步措施。
阅读全文
相关推荐
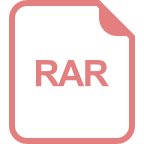
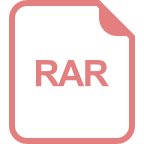
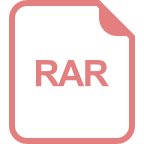
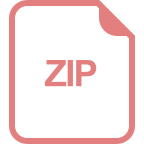
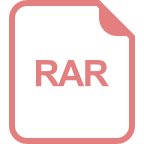
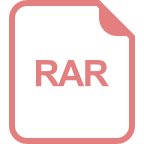
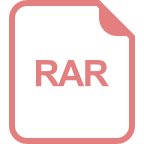
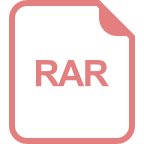
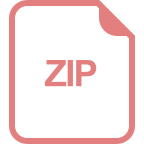
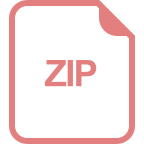
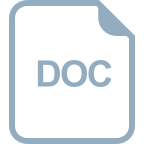
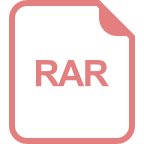
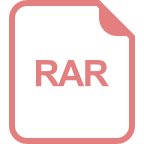
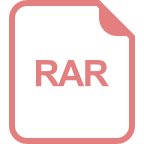
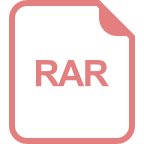
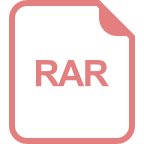
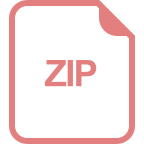