用c语言编写一个程序,实现环形队列的各种基本运算,队列的运输类型ElemType为char,并在此基础上设计一个主程序
时间: 2024-10-25 20:05:35 浏览: 29
在C语言中,我们可以使用数组和两个指针(一个用于指向队首元素,另一个用于指向队尾元素)来实现一个环形队列。这里是一个简单的环形队列结构和一些基本操作的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_SIZE 50
typedef char ElemType;
struct Queue {
int front; // 队头索引
int rear; // 队尾索引
ElemType queue[MAX_SIZE];
};
// 创建新队列
Queue* createQueue() {
Queue* q = (Queue*) malloc(sizeof(Queue));
if (!q) {
printf("Memory allocation failed.\n");
return NULL;
}
q->front = -1;
q->rear = -1;
return q;
}
// 判断队列是否为空
int isEmpty(Queue* q) {
return q->front == -1 && q->rear == -1;
}
// 判断队列是否已满
int isFull(Queue* q) {
return (q->rear + 1) % MAX_SIZE == q->front;
}
// 入队
void enqueue(Queue* q, char elem) {
if (isFull(q)) {
printf("Queue is full.\n");
return;
}
if (isEmpty(q)) {
q->front = q->rear = 0;
} else {
q->rear = (q->rear + 1) % MAX_SIZE;
}
q->queue[q->rear] = elem;
}
// 出队
char dequeue(Queue* q) {
if (isEmpty(q)) {
printf("Queue is empty.\n");
return '\0';
}
char elem = q->queue[q->front];
if (q->front == q->rear) { // 如果队列只剩下一个元素
q->front = q->rear = -1;
} else {
q->front = (q->front + 1) % MAX_SIZE;
}
return elem;
}
// 主程序演示
int main() {
Queue* myQueue = createQueue();
// ... 进行入队、出队操作
enqueue(myQueue, 'A');
enqueue(myQueue, 'B');
printf("%c dequeued.\n", dequeue(myQueue)); // 输出 B
free(myQueue);
return 0;
}
```
这个程序实现了创建队列、判断队列状态、入队和出队的基本操作。在主程序中,你可以根据需要添加更多的队列操作,如显示队列内容等。
阅读全文
相关推荐
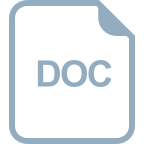
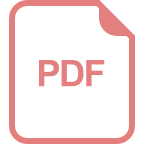
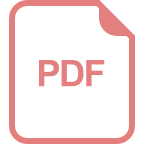


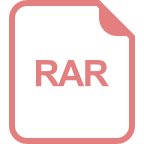
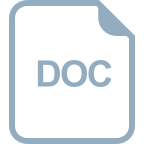
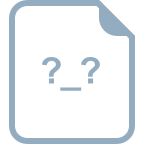
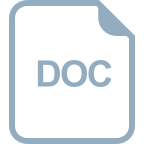
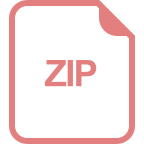
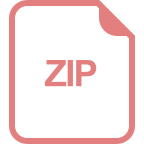
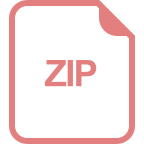
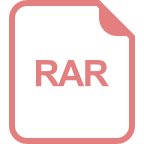
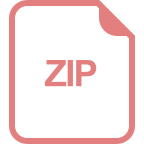
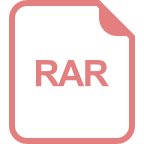