<router-view/>可单独使用吗
时间: 2024-06-14 14:08:23 浏览: 121
<router-view/>是Vue.js中的一个内置组件,用在单页面应用程序(SPA)中显示匹配到的路由组件。它通常与Vue Router一起使用,用于动态染匹配到的组件。
<router-view/>本身不能单独使用,它需要在Vue Router的配置中定义路由规则,并且需要在Vue实例中引入Vue Router插件。只有在这种情况下,<router-view/>才能正常工作。
在Vue Router的配置中,我们可以定义多个路由规则,每个规则对应一个组件。当用户访问不同的URL时,Vue Router会根据路由规则匹配到对应的组件,并将其渲染到<router-view/>中。
所以,<router-view/>是作为Vue Router的一部分来使用的,它负责根据当前路由的路径动态渲染对应的组件。
相关问题
<template> <div> <v-tabs class="tabs" fixed-tabs color="primary" v-model="tab"> <v-tab>招聘信息</v-tab> <v-tab> 简历 </v-tab> </v-tabs> <keep-alive> <router-view :key="$route.fullPath"></router-view> </keep-alive> </div></template><script>export default { data() { return { tab: 0, }; }, activated() { // Restore the last selected tab const savedTab = localStorage.getItem('activeTab'); if (savedTab) { this.tab = Number(savedTab); } }, beforeRouteLeave(to, from, next) { // Save the current selected tab before leaving localStorage.setItem('activeTab', this.tab); next(); },};</script>改为组合式
将Vue的选项式组件转换为组合式组件的过程需要注意一些细节,但大体上可以按照以下步骤进行:
1. 将模板代码移动到一个单独的.vue文件中,并将其命名为Tabs.vue。
2. 在Tabs.vue文件中,将模板代码包裹在一个template标签中,并将v-model指令绑定到一个名为“modelValue”的prop。这个prop将用于在组合式组件中跟踪当前选中的选项卡。
```
<template>
<div>
<v-tabs class="tabs" fixed-tabs color="primary" v-model="modelValue">
<v-tab>招聘信息</v-tab>
<v-tab> 简历 </v-tab>
</v-tabs>
<keep-alive>
<slot :key="$route.fullPath"></slot>
</keep-alive>
</div>
</template>
```
3. 在同一个Tabs.vue文件中,将data选项替换为一个setup函数,并将其中的数据属性“tab”替换为一个ref对象“modelValue”。
```
<script>
import { ref, onActivated, onBeforeRouteLeave } from 'vue';
export default {
props: {
modelValue: {
type: Number,
default: 0,
},
},
setup(props) {
const modelValue = ref(props.modelValue);
onActivated(() => {
const savedTab = localStorage.getItem('activeTab');
if (savedTab) {
modelValue.value = Number(savedTab);
}
});
onBeforeRouteLeave((to, from, next) => {
localStorage.setItem('activeTab', modelValue.value);
next();
});
return {
modelValue,
};
},
};
</script>
```
在这个setup函数中,我们首先使用Vue的ref函数创建一个名为“modelValue”的响应式对象,并将其初始值设置为从prop中传入的值。我们还使用Vue的onActivated和onBeforeRouteLeave钩子来处理组件的激活和离开事件。在onActivated钩子中,我们从本地存储中加载上次选定的选项卡,并将其设置为“modelValue”的值。在onBeforeRouteLeave钩子中,我们将当前选定的选项卡保存到本地存储中,以便在返回时恢复用户的选项卡选择。
最后,在模板中使用Vue的slot标签来定义插槽,以便在父组件中插入子组件。在这种情况下,我们使用了一个匿名插槽,将其绑定到一个名为“$route.fullPath”的动态key上,以便在切换路由时强制重新渲染子组件,以便正确恢复选项卡的状态。
将这个组合式组件在父组件中使用时,您可以像这样绑定modelValue属性:
```
<template>
<div>
<tabs v-model="activeTab">
<template #default>
<router-view />
</template>
</tabs>
</div>
</template>
<script>
import Tabs from './Tabs.vue';
export default {
components: {
Tabs,
},
data() {
return {
activeTab: 0,
};
},
};
</script>
```
在这个例子中,我们将Tabs组件导入到父组件中,并将其注册为局部组件。然后,我们使用v-model指令将父组件的“activeTab”变量绑定到组件的“modelValue”prop上。在组件内部,选项卡的状态将由“modelValue”ref对象跟踪和管理。
<template> <div> <router-view /> <div class="title">购物车</div> <div class="shop_goods" v-for="(item, index) in shopcarList" :key="index"> <van-checkbox v-model="item.isChecked"></van-checkbox> <van-card :title="item.name" :thumb="item.img"> <template #price> <span>¥{{ item.price }}</span> </template> <template #num> <p class="count"> <span @click="del(index)">-</span> <span>{{ item.num }}</span> <span @click="add(index)">+</span> </p> </template> </van-card> </div> <div class="shopcar_total"> <van-submit-bar :price="priceTotal" @submit="onSubmit"> <template #button> <span class="total"> 去结算 </span> </template> <van-checkbox v-model="isAllChecked">全选</van-checkbox> </van-submit-bar> </div> </div> </template> <script> export default { data() { return { stepper: 1, shopcarList: [], }; }, methods: { onSubmit() { console.log(111); }, add(index) { // console.log(index); this.$set( this.shopcarList[index], this.shopcarList[index].num, this.shopcarList[index].num++ ); }, del(index) { if (this.shopcarList[index].num == 1) { this.$toast("不能再减了"); } else { this.$set( this.shopcarList[index], this.shopcarList[index].num, this.shopcarList[index].num-- ); } }, }, created() { this.shopcarList = JSON.parse(localStorage.getItem("shopcar")) || []; console.log(this.shopcarList); }, computed: { isAllChecked: { set(val) { this.shopcarList.forEach((ele) => { ele.isChecked = val; }); }, get() { return this.shopcarList.every((ele) => { return ele.isChecked == true; }); }, }, priceTotal() { let sum = 0; let newPrice = this.shopcarList.filter((ele) => { return ele.isChecked == true; }); sum = newPrice.reduce((pre, current) => { return (pre += current.price * current.num); }, 0); return sum * 100; }, }, }; </script>这个页面怎么优化
这个页面可以优化的地方有:
1. 使用vue的keep-alive标签,对于需要频繁切换的组件进行缓存,减少重复渲染。
2. 使用v-show代替v-if,因为v-if每次都会销毁和重新创建DOM元素,而v-show只是控制元素的显示和隐藏。
3. 对于频繁使用的方法,可以将其提取为公共方法,避免重复代码。
4. 对于localStorage的操作,可以将其封装为一个单独的模块,并进行数据的有效性验证,避免出现数据异常。
5. 对于复杂的计算属性,可以使用缓存机制,避免重复计算。
6. 对于数组的修改,可以使用Vue提供的set方法,确保数据的响应式更新。
7. 可以考虑使用分页或懒加载等技术,对于大量数据进行分批加载,减轻页面的渲染压力。
8. 对于一些不必要的DOM元素,可以使用v-if或v-show进行控制,避免无意义的渲染。
9. 对于一些常用的组件,可以考虑进行全局注册或按需加载,避免重复引入和渲染。
阅读全文
相关推荐
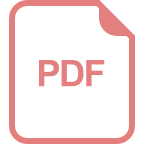
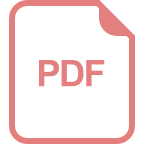




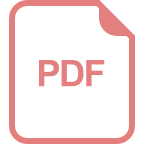
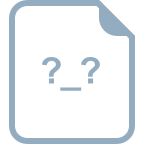
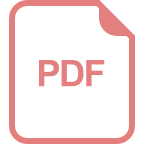
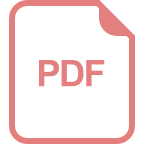
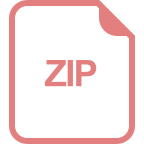
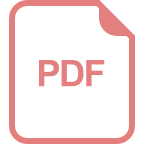
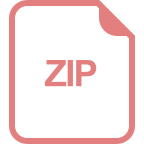
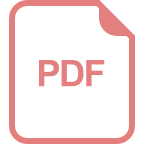
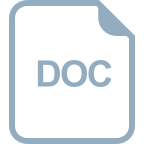
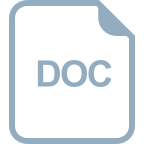