使用PyQt5创建一个FTP客户端应用程序。它连接到linux创建的FTP服务器,并提供了上传和下载文件的功能。
时间: 2024-04-11 15:33:49 浏览: 121
你可以使用PyQt5和ftplib库来创建一个FTP客户端应用程序。以下是一个简单的示例代码,可以连接到Linux上的FTP服务器并提供上传和下载文件的功能:
```python
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QLabel, QPushButton, QFileDialog
from PyQt5.QtCore import Qt
from ftplib import FTP
class FTPClient(QMainWindow):
def __init__(self):
super().__init__()
self.initUI()
def initUI(self):
self.setWindowTitle("FTP Client")
self.setGeometry(100, 100, 300, 200)
self.lbl_status = QLabel(self)
self.lbl_status.setAlignment(Qt.AlignCenter)
self.lbl_status.setGeometry(10, 10, 280, 30)
self.btn_upload = QPushButton("Upload", self)
self.btn_upload.setGeometry(10, 50, 130, 30)
self.btn_upload.clicked.connect(self.uploadFile)
self.btn_download = QPushButton("Download", self)
self.btn_download.setGeometry(150, 50, 130, 30)
self.btn_download.clicked.connect(self.downloadFile)
self.show()
def uploadFile(self):
file_path, _ = QFileDialog.getOpenFileName(self, "Select File to Upload")
if file_path:
ftp = FTP('ftp.example.com')
ftp.login('username', 'password')
with open(file_path, 'rb') as file:
ftp.storbinary('STOR ' + file_path.split('/')[-1], file)
ftp.quit()
self.lbl_status.setText("File uploaded successfully")
def downloadFile(self):
file_path, _ = QFileDialog.getSaveFileName(self, "Save File")
if file_path:
ftp = FTP('ftp.example.com')
ftp.login('username', 'password')
with open(file_path, 'wb') as file:
ftp.retrbinary('RETR ' + file_path.split('/')[-1], file.write)
ftp.quit()
self.lbl_status.setText("File downloaded successfully")
if __name__ == '__main__':
app = QApplication(sys.argv)
window = FTPClient()
sys.exit(app.exec_())
```
在代码中,你需要将`ftp.example.com`替换为你的FTP服务器的地址,`username`和`password`替换为你的登录凭据。上传和下载文件的功能分别在`uploadFile`和`downloadFile`方法中实现。
注意:这只是一个简单的示例,你可能需要添加错误处理和其他功能来完善你的FTP客户端应用程序。
阅读全文
相关推荐
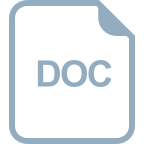
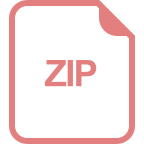
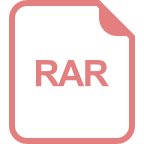
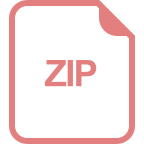
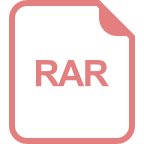
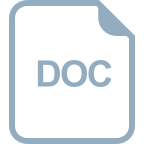
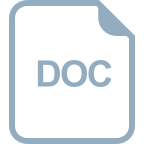
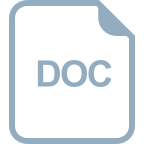
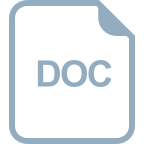
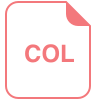

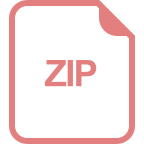
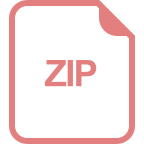