定义一个point类,输入10个点坐标。 ----计算出两两点之间的最短、最长距离并输出。 -----计算出10个点的重心坐标。 用c++完成上述操作。
时间: 2024-10-18 07:19:31 浏览: 55
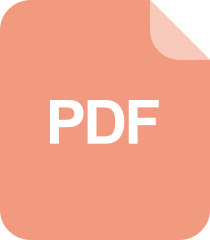
基于Levenbery-Marquardt算法的点衍射三维坐标测量方法
为了完成这个任务,我们可以定义一个`Point`类,包含x和y坐标,并提供两个成员函数,一个用于计算两点间的距离,另一个用于计算所有点的重心。首先,让我们定义`Point`类:
```cpp
#include <iostream>
#include <cmath>
#include <vector>
class Point {
private:
double x;
double y;
public:
// 构造函数
Point(double xCoord, double yCoord)
: x(xCoord), y(yCoord) {}
// 计算两点间距离
double distance(const Point &other) const {
double dx = other.x - x;
double dy = other.y - y;
return std::sqrt(dx * dx + dy * dy);
}
// 读取坐标
void inputCoordinates() {
std::cout << "请输入第" << ++pointCount << "个点的坐标 (x,y): ";
std::cin >> x >> y;
}
// 计算10个点的重心坐标
static Point calculateCentroid(std::vector<Point> points) {
double totalX = 0, totalY = 0;
for (const auto &point : points) {
totalX += point.x;
totalY += point.y;
}
return Point(totalX / points.size(), totalY / points.size());
}
};
int main() {
const int numPoints = 10;
Point points[numPoints];
int pointCount = 0;
for (int i = 0; i < numPoints; ++i) {
points[i].inputCoordinates();
}
// 计算任意两点间的最短和最长距离
std::cout << "两点间最短距离: ";
double shortestDistance = points[0].distance(points[1]);
for (int i = 1; i < numPoints; ++i) {
for (int j = i + 1; j < numPoints; ++j) {
double currentDistance = points[i].distance(points[j]);
if (currentDistance < shortestDistance) {
shortestDistance = currentDistance;
}
}
}
std::cout << shortestDistance << std::endl;
std::cout << "两点间最长距离: ";
double longestDistance = shortestDistance;
for (int i = 1; i < numPoints; ++i) {
for (int j = i + 1; j < numPoints; ++j) {
if (points[i].distance(points[j]) > longestDistance) {
longestDistance = points[i].distance(points[j]);
}
}
}
std::cout << longestDistance << std::endl;
// 计算所有点的重心坐标
Point centroid = Point::calculateCentroid(points);
std::cout << "重心坐标: (" << centroid.x << ", " << centroid.y << ")" << std::endl;
return 0;
}
```
这个程序首先定义了一个`Point`类,接着在`main`函数中创建了10个`Point`对象并输入它们的坐标。然后计算任意两点间的最短和最长距离,最后计算所有点的重心坐标。注意,在实际应用中,你可能会选择动态内存分配而不是数组,但在这种情况下,由于我们知道有固定数量的点,所以数组是可行的。
阅读全文
相关推荐
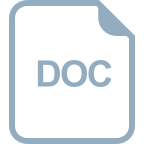
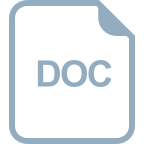
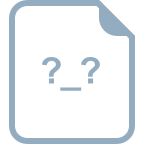
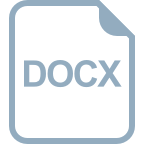
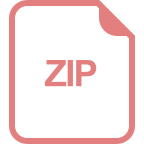
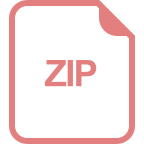
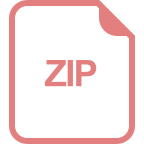
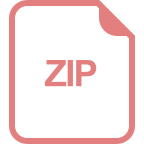
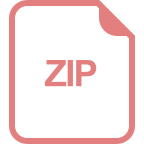
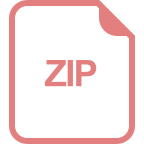
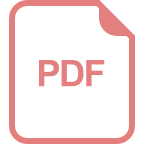
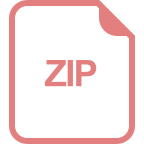
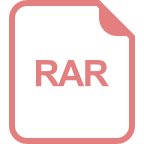
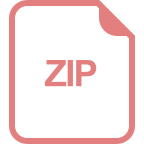
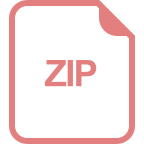