编写一个C程序,模拟银行转账操作,使用线程和同步机制确保操作的原子性和一致性
时间: 2024-10-21 19:13:14 浏览: 45
要编写一个C程序模拟银行转账操作,我们首先要了解线程同步的关键概念——原子性(保证单个操作不可分割)和一致性(确保操作完成后状态不变)。这里我们使用`pthread`库来实现线程并发控制。下面是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <pthread.h>
// 定义银行账户结构体
typedef struct {
long account;
} BankAccount;
// 初始化银行账户
BankAccount* init_account(long balance) {
BankAccount acc;
acc.account = balance;
return &acc;
}
// 转账函数,确保操作原子性
void transfer(BankAccount* from, BankAccount* to, long amount) {
pthread_mutex_t lock = PTHREAD_MUTEX_INITIALIZER; // 创建互斥锁
pthread_mutex_lock(&lock); // 锁定资源
from->account -= amount;
to->account += amount;
printf("Transfer of %ld completed.\n", amount);
pthread_mutex_unlock(&lock); // 解锁资源
}
int main() {
BankAccount src = init_account(100), dst = init_account(200);
pthread_t thread1, thread2;
// 创建两个线程
if (pthread_create(&thread1, NULL, &transfer, &src) != 0 ||
pthread_create(&thread2, NULL, &transfer, &dst) != 0) {
perror("Thread creation failed");
exit(EXIT_FAILURE);
}
// 等待线程结束
pthread_join(thread1, NULL);
pthread_join(thread2, NULL);
printf("Final balances:\n");
printf("Source: %ld\n", src.account);
printf("Destination: %ld\n", dst.account);
return 0;
}
```
在这个示例中,我们使用`pthread_mutex_lock`和`pthread_mutex_unlock`来保证转账操作的原子性,确保每次只有一个线程能访问账户余额。这样就避免了并发情况下可能出现的数据不一致。
阅读全文
相关推荐





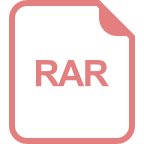


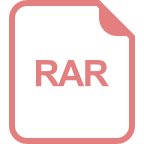









