如何用C++编程实现一个简单的小猿口算自动答题系统?
时间: 2024-10-17 16:03:59 浏览: 117
在C++中,实现一个小猿口算自动答题系统可以分为几个步骤:
1. **设计数据结构**:首先需要定义一些基本的数据结构来存储题目和答案,比如`Question`类,包含题面、选项和正确答案。
```cpp
class Question {
public:
int question;
std::vector<int> options;
int correctAnswer;
};
```
2. **用户输入处理**:创建函数接收用户的输入,例如口头表达的数学运算,通过解析用户语音或文字转换成整数运算。
```cpp
std::string userInput = "十加十五"; // 模拟用户输入
int operation, num1, num2;
parseUserInput(userInput, operation, num1, num2);
```
(这里`parseUserInput`是一个假设的函数,实际需要实现将字符串转为操作符和数字的功能)
3. **计算和判断答案**:根据用户提供的运算,计算结果并与预设的答案进行比较。
```cpp
int result = performOperation(operation, num1, num2); // 模拟实际运算
bool isCorrect = result == question.correctAnswer;
```
4. **反馈结果**:最后返回是否答对,并可能提供下一步的操作提示。
```cpp
if (isCorrect) {
std::cout << "答对了!" << std::endl;
} else {
std::cout << "错误,正确答案是:" << question.correctAnswer << std::endl;
}
```
5. **循环与控制流程**:为了实现持续的答题过程,可以在一个循环中不断获取用户输入并进行解答。
```cpp
while (true) {
// 用户答题...
}
```
阅读全文
相关推荐
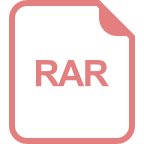
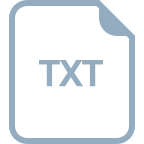
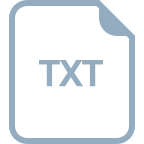
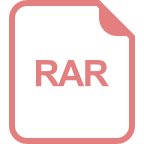
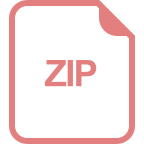
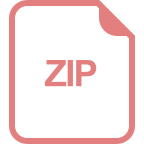
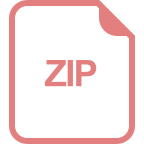
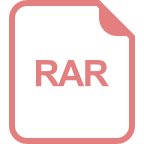
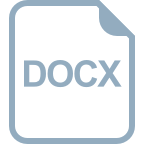
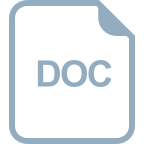
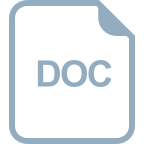
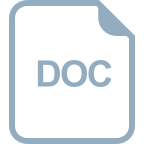
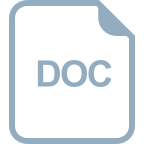
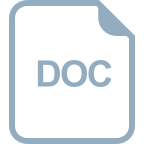