C#FTP自动下载文件
时间: 2024-10-07 12:02:23 浏览: 31
在C#中,如果你想要编写一个FTP自动下载文件的脚本,可以使用定时任务或者循环来定期访问FTP服务器。下面是一个基本的例子,展示了如何使用Task异步下载,并且设置一个间隔时间重复下载:
```csharp
using System;
using System.IO;
using System.Net;
using System.Threading.Tasks;
using System.Timers;
class FTPAutoDownloader {
private readonly string _ftpServerUrl;
private readonly string _username;
private readonly string _password;
private readonly string _remoteDirectory;
private readonly string _localPath;
private Timer _downloadTimer;
public FTPAutoDownloader(string ftpServerUrl, string username, string password, string remoteDirectory, string localPath) {
_ftpServerUrl = ftpServerUrl;
_username = username;
_password = password;
_remoteDirectory = remoteDirectory;
_localPath = localPath;
_downloadTimer = new Timer(60 * 1000); // 每分钟执行一次下载任务
_downloadTimer.Elapsed += OnDownloadTimerElapsed;
_downloadTimer.Enabled = true;
}
private void OnDownloadTimerElapsed(object sender, ElapsedEventArgs e) {
DownloadFilesFromFTP();
}
private async Task DownloadFilesFromFTP() {
try {
var directoryInfo = new DirectoryInfo(_ftpServerUrl + _remoteDirectory);
foreach (var fileInfo in directoryInfo.GetFiles()) {
var fileName = fileInfo.Name;
var filePath = Path.Combine(_localPath, fileName);
await DownloadFileAsync(fileInfo.FullName, filePath);
}
} catch (Exception ex) {
Console.WriteLine($"FTP下载失败: {ex.Message}");
}
}
private async Task DownloadFileAsync(string remoteFilePath, string localFilePath) {
using (FtpWebRequest request = (FtpWebRequest)WebRequest.Create(remoteFilePath)) {
request.Method = WebRequestMethods.Ftp.DownloadFile;
request.Credentials = new NetworkCredential(_username, _password);
using (var response = (FtpWebResponse)await request.GetResponseAsync()) {
await response.Stream.CopyToAsync(File.OpenWrite(localFilePath));
}
}
}
}
// 启动下载器
FAutoDownloader downloader = new FTPAutoDownloader("ftp://example.com", "your_username", "your_password", "/path/to/files/", "C:\\YourFolder\\Downloads");
```
在这个例子中,每隔一分钟(通过Timer控制),它会尝试从指定的FTP目录下载所有文件。
阅读全文
相关推荐
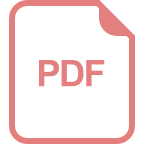
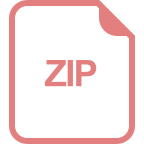
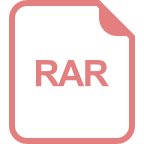
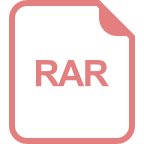
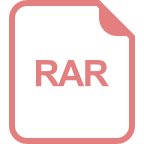
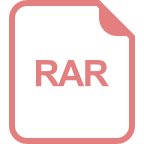
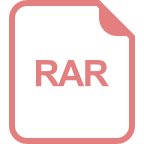
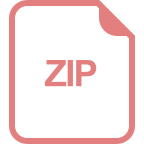
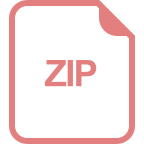
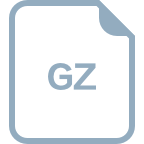
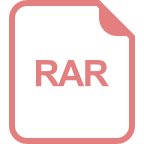
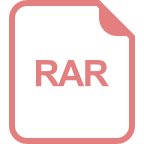
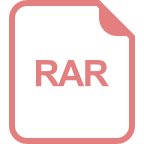
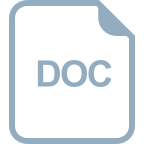
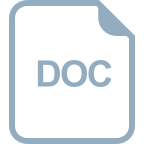
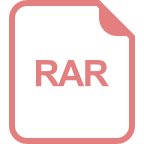
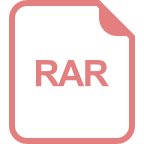
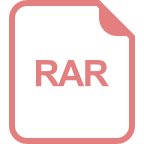
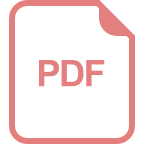