base_path = 'C: /wangzhao/Python/project'我现在有这个base_path,我还要定义一个在这个目录下的test1的目录,用python怎么写
时间: 2024-10-10 10:05:25 浏览: 14
在Python中,你可以使用`os.path`模块结合字符串操作来构建完整的目录路径。假设你已经有了`base_path`,你想在其中添加名为`test1`的新目录,可以这样做:
```python
import os
# 定义初始路径
base_path = 'C:/wangzhao/Python/project'
# 定义新的子目录名
new_directory = 'test1'
# 构建新路径
full_path = os.path.join(base_path, new_directory)
# 使用os.makedirs()创建目录,如果不存在则会自动创建
try:
os.makedirs(full_path)
except FileExistsError:
print(f"Directory {full_path} already exists.")
print(f"The full path to the 'test1' directory is: {full_path}")
```
这段代码首先使用`os.path.join()`函数将`base_path`和`new_directory`连接起来,形成完整的路径。然后使用`os.makedirs()`尝试创建该目录,如果目录已存在,则捕获可能出现的`FileExistsError`异常。
相关问题
打印一下test1目录下的所有文件的文件名
为了打印`test1`目录下的所有文件名,你需要先确保`test1`目录存在,并使用Python的标准库`os`和`glob`来获取目录内容。这里是一个示例:
```python
import os
import glob
# 假设你之前已经设置了base_path和test1目录
base_path = 'C:/wangzhao/Python/project'
test1_dir = os.path.join(base_path, 'test1')
# 检查test1目录是否存在
if not os.path.exists(test1_dir):
print(f"'{test1_dir}' does not exist.")
else:
# 获取test1目录下所有的文件(包括子目录)
file_list = glob.glob(os.path.join(test1_dir, '*'))
for filename in file_list:
if os.path.isfile(filename): # 验证是否为文件,排除可能的子目录
print("File:", filename)
```
这段代码首先检查`test1`目录是否存在,然后使用`glob.glob()`获取指定目录及其子目录下的所有文件。`'*'`是一个通配符,表示匹配任意文件。对于找到的每一个文件,再次确认它是文件而不是目录(使用`os.path.isfile()`),然后打印其名称。
1. 使用jQuery实现一个基本动画
好的,为您提供一个使用jQuery实现基本动画的例子:
例如,让一个元素向右移动:
```html
<!DOCTYPE html>
<html>
<head>
<title>jQuery动画实现</title>
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<style type="text/css">
#box {
width: 100px;
height: 100px;
background-color: blue;
position: relative;
left: 0;
}
</style>
</head>
<body>
<div id="box"></div>
<script type="text/javascript">
$(document).ready(function(){
$("#box").click(function(){
$(this).animate({left: '+=50px'}, 500);
});
});
</script>
</body>
</html>
```
这个例子中,我们定义了一个宽高为100px的蓝色方块,并且设置了它的初始位置为左侧边界。当用户点击方块时,使用jQuery的animate()方法实现一个向右移动50px的动画,并且动画时间为0.5秒。
阅读全文
相关推荐
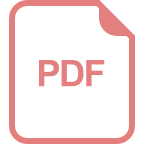
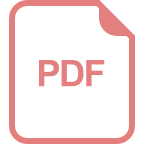
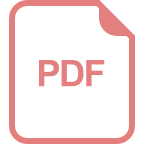
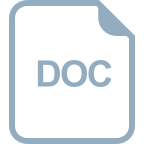
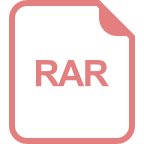
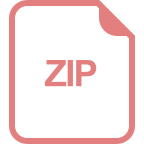
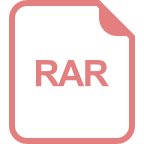
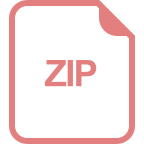
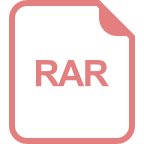
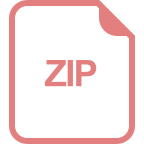
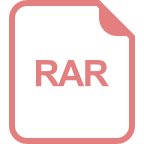
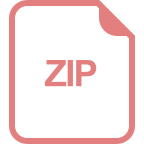
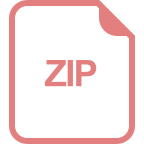
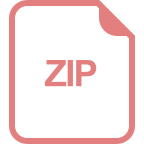