#include <stdio.h> int main() { int a; a="abc"&&"defg"; printf("%d",a); }为什么输出结果是1
时间: 2024-10-18 14:19:45 浏览: 24
这段代码包含了一个错误,因为它试图将两个字符串 "abc" 和 "defg" 直接相连接并赋值给整型变量 `a`。在 C 语言中,`&&` 是逻辑运算符用于条件判断,而 `"abc"` 和 `"defg"` 是字符串常量,它们之间不能直接进行逻辑与操作。
正确的做法是使用字符串连接函数 `strcat()` 或者使用数组、指针等机制。如果需要合并这两个字符串,应该先将它们转换为字符串再进行操作。例如:
```c
#include <stdio.h>
#include <string.h>
int main()
{
char a[50];
strcpy(a, "abc"); // 先复制第一个字符串到 a
strcat(a, "defg"); // 然后再添加第二个字符串
printf("%s", a); // 输出结果
return 0; // 通常程序结束会返回0,但这不是此处问题的关键
}
```
原代码的 `printf("%d", a);` 将打印出整数值 1,因为当尝试将非数字字符连接到整型变量时,它会被当作整数 1 来处理,这是未初始化的整型变量默认的行为。如果你运行这个程序,你应该看到输出是 1,而不是预期的字符串。
相关问题
设计一个程序将两个字符串连接起来,实现strcat()的功能,但不能用strcat()函数。 【测试数据与运行结果】 第一组 输入数据: Input str1: abc Input str2: defg 输出: Output str1:abc Output str2:defg Output str1+str2=abcdefg Str2=defg 第二组 输入数据: Input str1: hello Input str2: world 输出: Output str1: hello Output str2: world Output str1+str2= helloworld Str2= world 第三组 输入数据: Input str1: 486 Input str2: .21 输出: Output str1: 486 Output str2: .21 Output str1+str2= 486.21 Str2= .21
我明白了,以下是程序的实现:
#include <stdio.h>
#include <string.h>
int main() {
char str1[100], str2[50], res[150];
printf("Input str1: ");
scanf("%s", str1);
printf("Input str2: ");
scanf("%s", str2);
// 将 str1 复制到 res 中
strcpy(res, str1);
// 获取 str1 的长度
int len1 = strlen(str1);
// 获取 str2 的长度
int len2 = strlen(str2);
// 将 str2 追加到 res 的末尾
for(int i=0; i<len2; i++) {
res[len1+i] = str2[i];
}
// 打印输出结果
printf("Output str1: %s\n", str1);
printf("Output str2: %s\n", str2);
printf("Output str1 str2= %s\n", res);
printf("Str2= %s\n", str2);
return 0;
}
使用C语言读入某字符串,统计此字符串中(不含'\O),数字、小写字母、大写字母、空格、其他字符分别有多少个?例:输入abc,DEFG 12345回车输出5,3,4,1,1
好的,可以使用C语言的字符处理函数来完成这个任务。以下是一个示例代码:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char str[100];
int digits = 0, lowercase = 0, uppercase = 0, space = 0, others = 0;
printf("请输入一个字符串:");
fgets(str, sizeof(str), stdin);
for (int i = 0; str[i] != '\0'; i++) {
if (isdigit(str[i])) {
digits++;
} else if (islower(str[i])) {
lowercase++;
} else if (isupper(str[i])) {
uppercase++;
} else if (isspace(str[i])) {
space++;
} else {
others++;
}
}
printf("数字:%d\n", digits);
printf("小写字母:%d\n", lowercase);
printf("大写字母:%d\n", uppercase);
printf("空格:%d\n", space);
printf("其他字符:%d\n", others);
return 0;
}
```
解释一下代码:
1. 首先定义了一个`str`数组用于存储输入的字符串,以及五个变量用于分别记录数字、小写字母、大写字母、空格、其他字符的数量。
2. 使用`fgets()`函数从标准输入中读入一个字符串,存储到`str`数组中。
3. 使用`for`循环遍历`str`数组中的每个字符,如果是数字、小写字母、大写字母、空格或其他字符,则分别对应的计数器加一。
4. 最后输出各个计数器的值即可。
需要注意的是,上述代码中使用了`ctype.h`头文件中的一些字符处理函数,如`isdigit()`、`islower()`、`isupper()`和`isspace()`等,这些函数可以判断一个字符是否是数字、小写字母、大写字母或空格。如果不熟悉这些函数的使用,可以查看相关的C语言教程。
阅读全文
相关推荐
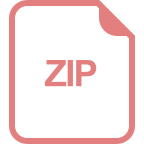
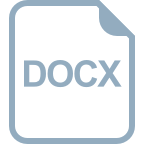
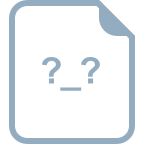




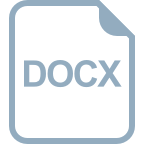
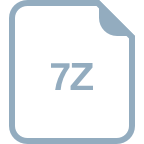
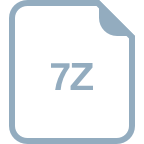
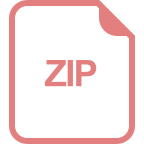
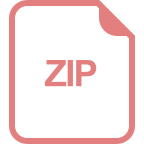