随机森林特征重要性python
时间: 2023-11-13 17:57:57 浏览: 132
好的,关于随机森林特征重要性的问题,你可以使用sklearn库中的RandomForestRegressor或RandomForestClassifier模型来计算特征重要性。具体步骤如下:
1. 导入需要的库和数据集
```python
from sklearn.ensemble import RandomForestRegressor
import pandas as pd
data = pd.read_csv('your_data.csv')
X = data.drop('target', axis=1)
y = data['target']
```
2. 训练随机森林模型并计算特征重要性
```python
rf = RandomForestRegressor(n_estimators=100, random_state=42)
rf.fit(X, y)
importances = rf.feature_importances_
```
3. 可视化特征重要性
```python
import matplotlib.pyplot as plt
feat_importances = pd.Series(importances, index=X.columns)
feat_importances.nlargest(10).plot(kind='barh')
plt.show()
```
这里的n_estimators是指随机森林中树的数量,random_state是随机种子,用于保证每次运行结果一致。
相关问题
python随机森林特征重要性
在 Python 中,可以使用 scikit-learn 库中的 RandomForestRegressor 或 RandomForestClassifier 类来训练随机森林模型,并通过 feature_importances_ 属性来获取特征重要性。
以 RandomForestRegressor 为例,可以按以下步骤计算特征重要性:
1. 导入库和数据集:
```python
from sklearn.ensemble import RandomForestRegressor
import numpy as np
# 导入数据集
X = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9], [10, 11, 12]])
y = np.array([1, 2, 3, 4])
```
2. 训练随机森林模型:
```python
rf = RandomForestRegressor(n_estimators=100, random_state=42)
rf.fit(X, y)
```
其中,n_estimators 参数表示树的数量,random_state 参数用于设置随机种子,以确保可重复性。
3. 获取特征重要性:
```python
importances = rf.feature_importances_
```
4. 可视化特征重要性:
```python
import matplotlib.pyplot as plt
# 可视化特征重要性
plt.bar(range(X.shape[1]), importances)
plt.xticks(range(X.shape[1]), ['feat1', 'feat2', 'feat3'])
plt.show()
```
这将生成一个柱状图,其中 X 轴表示特征,Y 轴表示特征重要性得分。
需要注意的是,特征重要性得分并不总是准确的,因为它们可能会受到数据集中其他特征的影响。此外,特征重要性得分仅适用于当前训练数据集,不一定适用于其他数据集。
影像组学随机森林特征筛选的python代码
影像组学(Radiomics)是一种从医学图像中提取定量特征的技术,而随机森林(Random Forest)是一种常用的机器学习算法,常用于特征选择。在Python中,我们可以使用sklearn库中的RandomForestClassifier进行特征筛选。以下是一个简单的示例,展示如何使用随机森林进行特征重要性评估并筛选关键特征:
```python
# 导入所需的库
import numpy as np
from sklearn.ensemble import RandomForestClassifier
from sklearn.feature_selection import SelectFromModel
from skimage.io import imread
import os
# 假设你有已标记的训练数据集和对应图像文件
X_train = ... # 图像特征矩阵
y_train = ... # 训练标签
image_files = ... # 医学图像文件列表
# 构建随机森林分类器
clf = RandomForestClassifier(n_estimators=100, random_state=42)
# 使用随机森林训练模型
clf.fit(X_train, y_train)
# 通过随机森林的重要性得分对特征进行排序
feature_importances = clf.feature_importances_
# 创建特征选择器
selector = SelectFromModel(clf, prefit=True)
# 应用特征选择到训练数据
X_train_selected = selector.transform(X_train)
# 可能需要调整阈值来选取最重要的特征
threshold = np.percentile(feature_importances, 95) # 例如保留前95%重要的特征
selected_features = np.where(selector.get_support() == True)[0]
# 打印选定的特征索引
print("Selected features with importance above the threshold:")
print(selected_features)
#
阅读全文
相关推荐
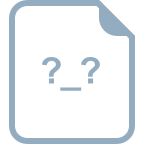













