Vue3+ts需要路由守卫的页面怎么写
时间: 2024-10-20 14:04:57 浏览: 53
在Vue3 + TypeScript项目中,使用路由守卫(也称为Guards)来控制用户的权限、处理路由跳转前后的操作是很常见的。首先,你需要安装Vue Router和@vue/cli-plugin-router的TypeScript插件支持。
1. 安装依赖:
```bash
npm install vue-router @vue/cli-plugin-router typescript --save
```
2. 创建一个通用的路由守卫类型:
```typescript
// router/guards.ts
import { RouteGuardOptions, Route } from 'vue-router';
type AuthRouteGuard = {
canActivate: (to: Route, from: Route, next: () => void) => boolean | Promise<boolean>;
async beforeEnter?(to: Route, from: Route, next: () => void): Promise<void> | void;
};
```
3. 配置全局守卫:
```typescript
// main.ts 或者 your-router-config.ts
import { createRouter, createWebHistory, use } from 'vue-router';
import { AuthRouteGuard } from './router/guards';
const routes: Array<Route> = ...; // 路由配置
const router = createRouter({
history: createWebHistory(process.env.BASE_URL),
routes,
guards: {
beforeEach((to, from, next) => {
const authGuard: AuthRouteGuard = to.meta?.auth || {} as AuthRouteGuard;
if (!authGuard.canActivate) return next(); // 如果没有设置canActivate,默认直接通过
authGuard.canActivate(to, from, async (resolve) => {
await someAuthCheck(); // 替换为实际的认证检查逻辑
resolve(true); // 认证通过则继续,失败则阻止进入
})(next);
}),
},
});
```
在这个例子中,`someAuthCheck`是你自定义的验证函数,可以检查用户是否登录等条件。每个需要权限保护的路由组件,在`meta`选项中添加`auth`字段,配置相应的守卫逻辑:
```typescript
// 组件文件
export default {
// ...
meta: {
auth: {
canActivate: async (to, from, next) => {
// 检查用户是否已经登录...
if (loggedIn) {
next();
} else {
next(false); // 返回false将停止导航到此路由
}
},
},
},
};
```
阅读全文
相关推荐
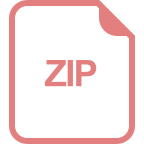
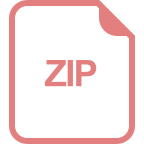
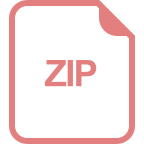








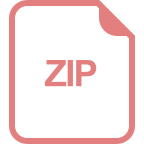
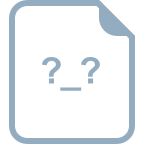
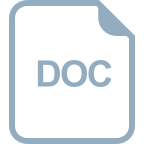




