输入字符串和字符,要求去掉中所有的字符,并输出结果。 可能出现全为空格的字符串和字符。
时间: 2024-09-30 21:14:18 浏览: 45
这个问题描述的是字符串的去除非指定字符的操作,通常在编程中通过遍历字符串并检查每个字符是否是我们想要保留的,然后将其添加到新的字符串中实现。例如,在Python中,可以这样做:
```python
def remove_char(input_str, char):
result = ""
for c in input_str:
if c != char:
result += c
return result
# 示例
input_string = "Hello, World!"
char_to_remove = ', '
output = remove_char(input_string, char_to_remove)
print(output) # 输出: Hello World!
# 对于全空格的情况,只要不是char,都会保留在结果中
empty_spaces = " "
output_empty = remove_char(empty_spaces, ' ')
print(output_empty) # 输出: (全为空格)
```
这里我们假设字符是指定的单个字符,如果是多个字符需要去除非指定集合内的所有字符。
相关问题
输入字符串和字符,要求去掉中所有的字符,并输出结果。 可能出现全为空格的字符串和字符。 输入格式: 测试数据有多组,每组输入字符串和字符。 输出格式: 对于每组输入,输出去除$$字符后的结果。如果结果为空,输出NULL。
在编程中,如果你需要从一个字符串中移除特定的字符,可以遍历字符串,检查每个字符是否是你想要移除的,如果不是,则将其添加到新的字符串中。以下是使用Python的一个简单示例:
```python
def remove_char(input_string, char_to_remove):
output = ""
for c in input_string:
if c != char_to_remove:
output += c
return output if output else "NULL"
# 测试数据
test_data = [("hello world", "l"), (" ", " ")]
for data in test_data:
result = remove_char(data[0], data[1])
print(f"去除'{data[1]}'后的结果:{result}")
```
这个函数会接收一个字符串`input_string`和一个字符`char_to_remove`,然后返回一个新的字符串,其中不含指定字符。如果处理后的结果是空字符串,就返回"NULL"。
输入字符串和字符,要求去掉中所有的字符,并输出结果。 可能出现全为空格的字符串和字符。 输入格式: 测试数据有多组,每组输入字符串和字符。 输出格式: 对于每组输入,输出去除$$字符后的结果。如果结果为空,输出NULL。用python写一段代码
当然可以,你可以使用Python的内建函数`str.replace()`来替换掉指定的字符。下面是一段示例代码,它接受两个参数——字符串和要移除的字符,然后返回新字符串:
```python
def remove_char(input_string, char_to_remove):
# 检查输入是否为空或只包含空格
if not input_string.strip():
return "NULL"
# 使用replace()函数移除字符
result = input_string.replace(char_to_remove, '')
return result
# 示例测试数据
test_data = [
(" ", "$$"),
("Hello $$ World", "$$"),
("$$", "$$"),
(" ", "$$"),
]
# 遍历测试数据并输出结果
for data in test_data:
output = remove_char(data[0], data[1])
print(f"去除'{data[1]}'后的结果: {output}")
```
这段代码首先检查输入字符串是否为空或只包含空格(通过`strip()`函数),如果是,则返回"NULL"。否则,使用`replace()`函数移除所有出现的指定字符,得到新的字符串。
如果你有其他特殊的需求,比如处理Unicode字符或者其他情况,请告诉我,我可以进一步调整代码。
阅读全文
相关推荐
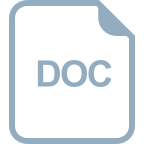
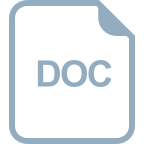
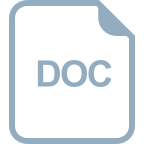









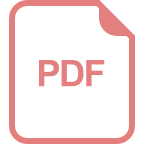
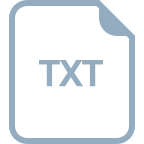
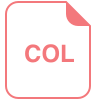

