linearlayout背景颜色 设置为null
时间: 2024-04-24 22:25:11 浏览: 11
如果您想将LinearLayout的背景颜色设置为null,即没有背景颜色,可以使用android:background属性并将其设置为"@null"。以下是示例代码:
```xml
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@null">
<!-- 在这里添加其他的视图元素 -->
</LinearLayout>
```
通过将android:background属性设置为"@null",可以将LinearLayout的背景颜色设置为透明,即没有任何背景颜色。
相关问题
android studio的linearlayout
Android Studio中的LinearLayout是一种用于在垂直或水平方向上排列子视图的布局容器。它可以根据指定的权重来分配子视图的空间。 在版本一中,LinearLayout的方向是垂直的,子视图按照顶部、中部和底部的顺序排列,并使用不同的背景颜色进行区分。在版本二中,LinearLayout的方向仍然是垂直的,但是使用了分隔线来分隔子视图,并通过dividerPadding属性设置了分隔线与子视图之间的间距。
如果你想在Android Studio中创建一个LinearLayout,你可以通过XML布局文件来定义它,并在其中添加子视图。你可以指定LinearLayout的方向、布局宽度和高度等属性,还可以设置权重来调整子视图的大小。此外,你还可以使用分隔线来美化LinearLayout的外观。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* [Android Studio —— LinearLayout](https://blog.csdn.net/automoblie0/article/details/122548137)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
- *3* [Android LinearLayout实现自动换行效果](https://download.csdn.net/download/weixin_38737366/14880886)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_2"}}] [.reference_item style="max-width: 50%"]
[ .reference_list ]
android画弧背景
要在 Android 中绘制弧形背景,可以使用 Canvas 和 Paint 类。以下是一个简单的示例:
1. 创建一个自定义 View 类,重写 onDraw() 方法。
```java
public class ArcBackgroundView extends View {
private Paint paint;
private RectF arcRectF;
public ArcBackgroundView(Context context) {
this(context, null);
}
public ArcBackgroundView(Context context, AttributeSet attrs) {
super(context, attrs);
init();
}
private void init() {
paint = new Paint();
paint.setColor(Color.BLUE);
paint.setStyle(Paint.Style.FILL);
paint.setAntiAlias(true);
arcRectF = new RectF();
}
@Override
protected void onDraw(Canvas canvas) {
super.onDraw(canvas);
int width = getWidth();
int height = getHeight();
arcRectF.left = 0;
arcRectF.top = 0;
arcRectF.right = width;
arcRectF.bottom = height;
canvas.drawArc(arcRectF, 0, 120, true, paint);
}
}
```
2. 在布局文件中添加自定义 View。
```xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<com.example.ArcBackgroundView
android:layout_width="match_parent"
android:layout_height="150dp"
/>
</LinearLayout>
```
这个示例绘制了一个蓝色的背景弧形,弧的起点是 0 度,终点是 120 度。可以根据需要调整颜色、起始角度和结束角度。
相关推荐
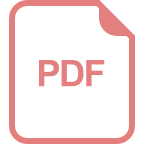
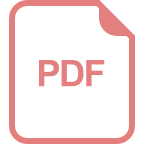











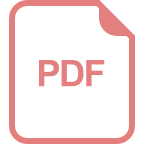