std::map遍历 c++17
时间: 2024-03-06 12:44:58 浏览: 28
在C++17中,可以使用范围for循环来遍历std::map容器。std::map是一个关联容器,它按照键值对的方式存储数据,并且按照键的顺序进行排序。
以下是使用范围for循环遍历std::map的示例代码:
```cpp
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap = {{1, "apple"}, {2, "banana"}, {3, "orange"}};
for (const auto& pair : myMap) {
std::cout << "Key: " << pair.first << ", Value: " << pair.second << std::endl;
}
return 0;
}
```
上述代码中,我们创建了一个std::map对象myMap,并初始化了一些键值对。然后,使用范围for循环遍历myMap,通过pair.first获取键,通过pair.second获取值,并输出到控制台。
相关问题
std::map遍历删除
在使用std::map遍历删除时,需要注意以下几点:
1.不要在遍历过程中直接删除元素,因为这会导致迭代器失效,可能会导致程序崩溃。
2.可以使用一个临时迭代器来保存下一个元素的位置,然后再删除当前元素。
3.可以使用C++11中的auto关键字和范围for循环来遍历std::map,这样可以避免手动管理迭代器。
下面是一个使用临时迭代器的示例代码:
```
std::map<std::string, Node>::iterator it_map, tmp;
std::vector<std::string>::iterator it_list;
std::string node_name;
for (it_map = node_map->begin(); it_map != node_map->end();) {
node_name = it_map->first;
it_list = std::find(node_list.begin(), node_list.end(), node_name);
if (it_list != node_list.end()) {
tmp = ++it_map;
node_map->erase(--it_map);
it_map = tmp;
} else {
++it_map;
}
}
```
这里使用了一个临时迭代器tmp来保存下一个元素的位置,然后再删除当前元素。注意,在删除元素之前需要将it_map减1,以便正确指向当前元素。
std::map 遍历
遍历 std::map 可以使用迭代器,示例代码如下:
```c++
#include <iostream>
#include <map>
int main() {
std::map<int, std::string> myMap = {{1, "one"}, {2, "two"}, {3, "three"}};
// 使用迭代器遍历
for (auto it = myMap.begin(); it != myMap.end(); ++it) {
std::cout << it->first << ": " << it->second << std::endl;
}
return 0;
}
```
输出结果为:
```
1: one
2: two
3: three
```
相关推荐
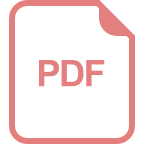
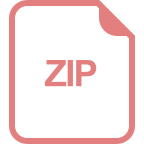
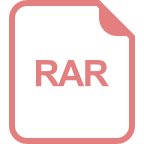












