用c++定义一个时间类,用来存储时,分,秒的信息,类中还有带参数的构造函数和显示时间的成员函数并且定义主函数测试设计的类
时间: 2024-09-09 22:00:39 浏览: 74
在C++中,你可以创建一个名为`Time`的时间类,它包含小时、分钟和秒三个私有变量,并提供相应的构造函数、赋值操作符以及一个用于显示时间的成员函数。以下是这个类的基本设计:
```cpp
#include <iostream>
class Time {
private:
int hours;
int minutes;
int seconds;
public:
// 构造函数,接受小时、分钟和秒作为参数
Time(int h = 0, int m = 0, int s = 0) : hours(h), minutes(m), seconds(s) {}
// 赋值运算符,用于设置时间
Time& setTime(int h, int m, int s) {
hours = h;
minutes = m;
seconds = s;
return *this;
}
// 显示时间的成员函数,通常会返回一个字符串形式的时间
std::string displayTime() const {
return std::to_string(hours) + ":" + std::to_string(minutes) + ":" + std::to_string(seconds);
}
// 主函数测试类的实例
static void mainFunction() {
Time currentTime(14, 30, 45); // 设置当前时间为下午2点30分45秒
std::cout << "Current time: " << currentTime.displayTime() << std::endl;
Time tomorrow(15, 0, 0); // 设置明天时间为下午3点
tomorrow.setTime(15, 0, 0); // 修改时间
std::cout << "Tomorrow's time: " << tomorrow.displayTime() << std::endl;
}
};
```
阅读全文
相关推荐











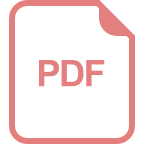

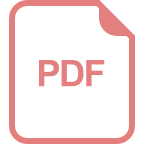
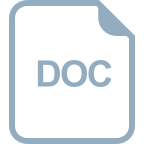



