向文件写入一串字符串。
时间: 2024-09-16 21:00:48 浏览: 33
在C++中,向文件写入一串字符串通常涉及以下几个步骤:
1. 首先,打开文件。可以使用`std::fstream`库中的`ofstream`类来完成。例如:
```cpp
#include <fstream>
#include <string>
std::ofstream file("example.txt", std::ios::app); // 'app'标志追加模式,避免覆盖原有内容
if (!file.is_open()) {
std::cerr << "Failed to open the file!" << std::endl;
return; // 处理打开失败的情况
}
```
2. 然后,调用文件流对象的`write()`或`<<`运算符来写入字符串。`write()`用于底层字节级操作,而`<<`则更为直观:
```cpp
std::string str = "Hello, World!";
file << str;
```
3. 最后,记得关闭文件以释放系统资源:
```cpp
file.close();
```
完整示例:
```cpp
#include <fstream>
#include <string>
int main() {
std::ofstream file("example.txt", std::ios::app);
if (!file.is_open()) {
std::cerr << "Failed to open the file!" << std::endl;
return 1;
}
std::string str = "This is a test string.\n";
file << str;
file.close(); // 关闭文件
return 0;
}
```
当你运行此程序,"example.txt" 文件将包含字符串 "This is a test string.\n"。每行结束符`\n`会在写入时自动添加。
阅读全文
相关推荐
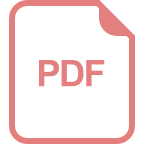
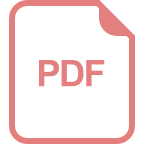
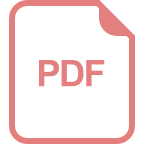
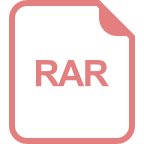
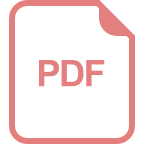
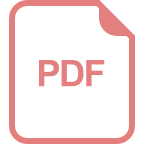
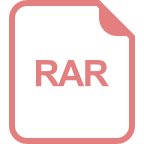
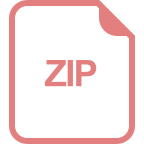
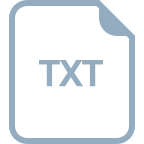
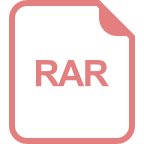
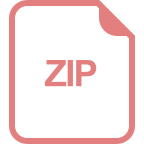
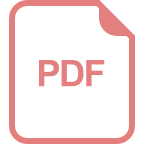
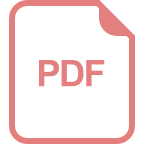
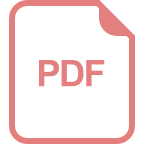
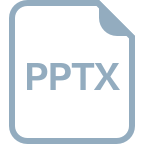
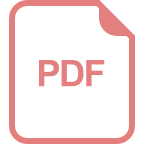
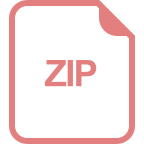
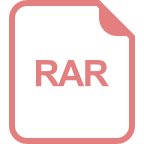
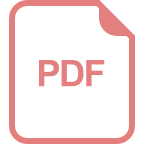