使用uni-popup组件来实现弹出层的效果并在其他页面点击按钮隐藏显示调用
时间: 2024-04-21 09:22:58 浏览: 115
要在其他页面上点击按钮来隐藏或显示弹出层,你可以使用 Vuex 来实现状态管理。以下是一个示例代码,展示了如何在不同页面上通过点击按钮来隐藏或显示弹出层:
首先,在你的 uni-app 项目中安装 Vuex:
```
npm install vuex
```
然后,创建一个 `store` 文件夹,并在该文件夹下创建一个 `index.js` 文件,用于创建和管理 Vuex 的状态。
store/index.js:
```javascript
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
export default new Vuex.Store({
state: {
showPopup: false
},
mutations: {
setShowPopup(state, payload) {
state.showPopup = payload
}
},
actions: {
setShowPopup({ commit }, payload) {
commit('setShowPopup', payload)
}
},
getters: {
showPopup(state) {
return state.showPopup
}
}
})
```
接下来,在需要显示弹出层的页面中,引入 `uni-popup` 组件和 Vuex 的 `store`:
your-page.vue:
```vue
<template>
<view>
<uni-popup :show="showPopup" @close="closePopup">
<!-- 弹出层的内容 -->
</uni-popup>
<!-- 其他页面内容 -->
<button @click="openPopup">打开弹出层</button>
</view>
</template>
<script>
import { mapGetters, mapActions } from 'vuex'
import uniPopup from '@dcloudio/uni-popup'
export default {
components: {
uniPopup
},
computed: {
...mapGetters(['showPopup'])
},
methods: {
...mapActions(['setShowPopup']),
openPopup() {
this.setShowPopup(true)
},
closePopup() {
this.setShowPopup(false)
}
}
}
</script>
```
在上述示例中,我们使用 Vuex 来管理 `showPopup` 的状态。在 `actions` 中,我们定义了一个 `setShowPopup` 的 action,用于改变 `showPopup` 的值。在页面中的 `openPopup` 方法中,我们调用 `setShowPopup(true)` 来显示弹出层;在 `closePopup` 方法中,我们调用 `setShowPopup(false)` 来隐藏弹出层。
在其他页面中,你可以通过点击按钮来改变 `showPopup` 的值,从而控制弹出层的显示和隐藏。只需要在点击按钮的事件中调用 `setShowPopup` action,并传递相应的参数即可。
记得根据实际情况调整代码,并确保在需要显示弹出层的页面中引入正确的组件和 Vuex 的 `store`。
阅读全文
相关推荐




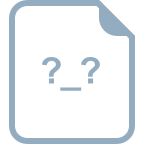










