c++判断字符串是否为指定字符串
时间: 2023-11-15 17:53:49 浏览: 54
判断一个字符串是否为回文有多种方法,以下是其中一种方法:
```c
#include <stdio.h>
#include <string.h>
int isPalindrome(char *str) {
int left = 0;
int right = strlen(str) - 1;
while (left < right) {
if (str[left] != str[right]) {
return 0;
}
left++;
right--;
}
return 1;
}
int main() {
char str[100];
printf("请输入一个字符串:");
scanf("%s", str);
if (isPalindrome(str)) {
printf("字符串是回文。\n");
} else {
printf("字符串不是回文。\n");
}
return 0;
}
```
相关问题
C++ 判断字符串的结尾是否是某个字符串
在Java中,可以使用String类的endsWith()方法来判断字符串的结尾是否是某个字符串。该方法返回一个boolean值,如果字符串以指定的字符串结尾,则返回true,否则返回false。
在给定的代码示例中,使用了endsWith()方法来判断字符串str1和str2的结尾是否分别为"?"和"。",并将结果打印出来。根据结果,可以看到字符串str1以"?"结尾,而字符串str2并不以"。"结尾。
因此,对于你的问题,C判断字符串的结尾是否是某个字符串,你可以使用Java的endsWith()方法进行判断。
C++ 判断字符串格式是否正确的快捷方式
可以使用正则表达式来判断字符串格式是否正确。C++11及以上版本提供了 `<regex>` 库,可以用来进行正则表达式匹配。
以下是一个示例代码,用于判断输入的字符串是否为合法的邮箱地址:
```c++
#include <iostream>
#include <regex>
int main() {
std::string email;
std::regex pattern("[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\\.[A-Za-z]{2,}");
std::cout << "请输入邮箱地址:";
std::getline(std::cin, email);
if (std::regex_match(email, pattern)) {
std::cout << "输入的邮箱地址合法" << std::endl;
} else {
std::cout << "输入的邮箱地址不合法" << std::endl;
}
return 0;
}
```
上述代码首先定义了一个正则表达式模式,然后使用 `std::regex_match()` 函数进行匹配。如果匹配成功,则说明输入的字符串符合指定的格式,否则不符合。
相关推荐
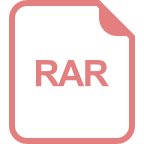
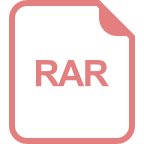












