用kotlin语言用volley框架写关于天气预报中的未来天气部分
时间: 2024-10-26 11:17:51 浏览: 39
在Kotlin中,Volley是一个强大的网络请求库,常用于Android应用中获取数据。要使用Volley来获取天气预报信息,首先需要添加Volley依赖,并创建一个简单的API请求来获取未来几天的天气预报。
1. 添加Volley依赖:
如果你使用的是Android Studio,可以在build.gradle文件的dependencies区块内添加以下依赖:
```groovy
implementation 'com.android.volley:volley:1.1.1'
```
2. 创建一个Volley RequestQueue实例:
```kotlin
val requestQueue = Volley.newRequestQueue(context)
```
3. 编写一个FutureWeatherService类,封装Volley请求:
```kotlin
class FutureWeatherService(private val apiKey: String) {
fun getWeatherForFutureDates(dates: List<String>, callback: (List<WeatherForecast>) -> Unit) {
dates.forEach { date ->
val url = "https://api.openweathermap.org/data/2.5/forecast?q=your_city_name&appid=$apiKey&units=metric&cnt=7&dt=${date.toLong()}"
val stringRequest = StringRequest(Request.Method.GET, url) {
responseString.use {
try {
val forecasts = parseWeatherResponse(response)
callback(forecasts)
} catch (e: Exception) {
// Handle error
}
}
}
requestQueue.add(stringRequest)
}
}
private fun parseWeatherResponse(response: String): List<WeatherForecast> {
// 解析JSON响应并创建WeatherForecast对象列表
// 使用Gson或者其他JSON解析库帮助你完成这一步
// 示例:
// val gson = Gson()
// return gson.fromJson(response, Array<WeatherForecast>::class.java).toList()
// 这里省略了具体的解析过程
TODO("Implement JSON parsing")
}
}
data class WeatherForecast(val date: Long, val description: String, val temperature: Double) // 假设这是从API返回的数据结构
```
4. 在Activity或Fragment中使用服务:
```kotlin
val futureWeatherService = FutureWeatherService(apiKey = "YOUR_API_KEY")
futureWeatherService.getWeatherForFutureDates(listOf("now", "tomorrow", "day_afterTomorrow"), { forecasts ->
forecasts.forEach { forecast ->
Log.d("Weather", "Date: ${forecast.date}, Description: $forecast.description, Temperature: $forecast.temperature")
// 在这里处理获取到的未来天气数据
}
})
```
注意:这个示例假设你有一个公开的API可以提供天气预报数据。你需要替换`"your_city_name"`为你实际查询的城市名,以及获取API密钥。此外,解析JSON的部分需要你自己编写或者使用第三方库来处理。
阅读全文
相关推荐
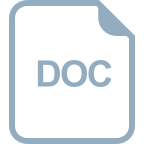
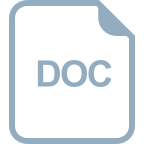
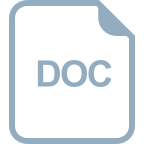
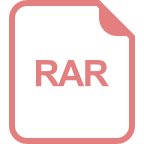
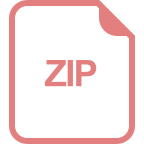
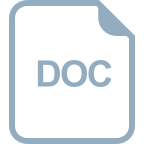
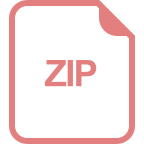
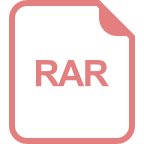
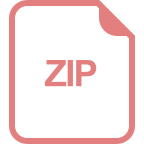
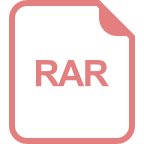
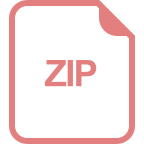
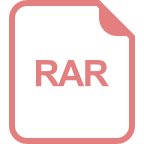
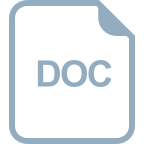
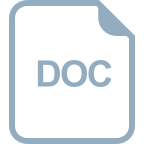
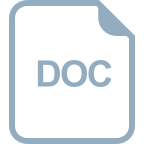
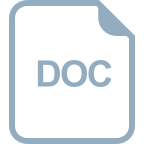
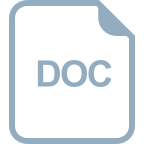
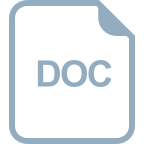
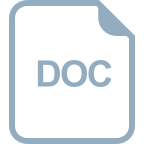