统计当前目录及所有子目录下,C 语言源文件的代码总行数
时间: 2024-09-25 14:03:34 浏览: 33
统计 C 语言源文件的代码总行数涉及到遍历文件系统并解析文件内容。在 C 语言中,你可以使用标准库函数如 `readdir` 和 `fgets` 来逐个读取目录中的文件名,然后递归地检查每个子目录。下面是一个简单的示例,展示了如何使用这些函数:
```c
#include <dirent.h>
#include <stdio.h>
#include <string.h>
// 函数来计算单个文件的行数
int count_lines(const char* filename) {
int line_count = 0;
FILE* file = fopen(filename, "r");
if (file) {
while (fgets(line, sizeof(line), file)) {
line[strcspn(line, "\n")] = '\0'; // 移除换行符
line_count++;
}
fclose(file);
}
return line_count;
}
// 主函数计算目录内所有 .c 文件的行数
size_t count_code_lines_in_dir(char* dir_path) {
size_t total_lines = 0;
DIR* dp = opendir(dir_path);
if (dp) {
struct dirent* entry;
while ((entry = readdir(dp))) {
if (strstr(entry->d_name, ".c")) { // 检查是否为.c文件
total_lines += count_lines(strcat(dir_path, "/", entry->d_name));
}
}
closedir(dp);
}
return total_lines;
}
int main() {
char path[] = "./"; // 修改为你想要统计的目录路径
size_t total_code_lines = count_code_lines_in_dir(path);
printf("Total lines of C source files in the directory: %zu\n", total_code_lines);
return 0;
}
```
请注意,这个示例假设所有的 C 语言源文件都是文本格式,并且不包含注释行。实际情况下,你可能需要更复杂的正则表达式或其他方法来准确计数代码行。
阅读全文
相关推荐
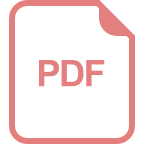
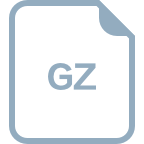
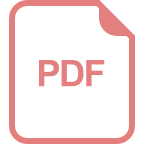
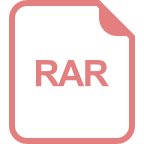
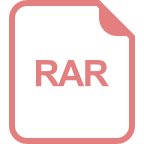
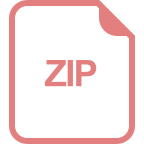
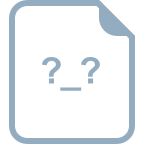
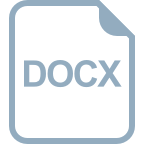
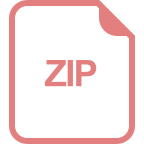
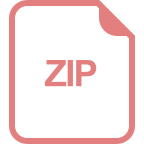
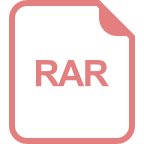