如何在OpenGL中实现基于扫描线算法的二维图形填充?请提供C++代码示例。
时间: 2024-11-19 15:37:26 浏览: 50
为了实现OpenGL中的扫描线算法,我们首先需要了解这一算法的基础概念和实现的关键步骤。扫描线填充算法通常用于图形库中绘制复杂多边形的内部区域填充,通过逐行扫描屏幕并处理边界线段来完成填充。在此过程中,活动边表(AET)和重要事件表(ET)是核心数据结构。
参考资源链接:[OpenGL扫描线填充算法实现与分析](https://wenku.csdn.net/doc/6id91fqsmz?spm=1055.2569.3001.10343)
下面是一个简化的C++代码示例,展示如何在OpenGL中使用扫描线算法进行图形填充。此示例重点在于算法的核心逻辑,不包含完整的OpenGL渲染流程代码,建议结合《OpenGL扫描线填充算法实现与分析》一文中的详细解释和代码实例进行学习。
```cpp
struct Line {
float x1, y1, x2, y2;
float dx() { return (x2 - x1) / (y2 - y1); }
Line(float x1, float y1, float x2, float y2) : x1(x1), y1(y1), x2(x2), y2(y2) {}
};
std::vector<Line> edges; // 存储所有线段的集合
std::map<float, std::vector<Line*>> activeEdges; // 活动边表(AET),按y坐标排序存储
// 初始化AET
void initAET() {
for (auto& edge : edges) {
if (edge.y1 == edge.y2) continue; // 跳过横线
activeEdges[edge.y1].push_back(&edge);
}
for (auto& pair : activeEdges) {
std::sort(pair.second.begin(), pair.second.end(), [](const Line* a, const Line* b) {
return a->dx() < b->dx();
});
}
}
// 扫描线填充算法的实现
void scanlineFill(float hmin, float hmax) {
for (float y = hmin; y <= hmax; ++y) {
// 获取当前扫描线的线段列表
auto it = activeEdges.lower_bound(y);
if (it != activeEdges.end()) {
if (it->first != y) {
it--;
}
for (auto& linePtr : it->second) {
// 这里填充算法的细节(如交点计算、扫描线填充等)
// ...
}
}
// 更新AET,移除已经结束的线段,添加新的线段
for (auto& pair : activeEdges) {
auto& lines = pair.second;
for (auto it = lines.begin(); it != lines.end();) {
if ((*it)->y1 == y) {
it = lines.erase(it); // 移除起点为当前y的线段
} else {
++it;
}
}
}
}
}
// 使用示例
int main() {
// 创建图形的线段集合
edges.emplace_back(1, 1, 5, 5);
edges.emplace_back(5, 1, 5, 5);
edges.emplace_back(1, 5, 5, 5);
edges.emplace_back(1, 1, 1, 5);
// 初始化AET并开始填充
initAET();
scanlineFill(1, 5);
// 其余OpenGL渲染代码...
}
```
请注意,这个代码示例需要结合具体的OpenGL环境和图形绘制流程,需要用户有一定的OpenGL编程基础。为了更深入理解扫描线算法在OpenGL中的应用,建议阅读《OpenGL扫描线填充算法实现与分析》,该资料不仅包含上述代码的详细解释,还有完整的示例代码和更深层次的图形学知识。
参考资源链接:[OpenGL扫描线填充算法实现与分析](https://wenku.csdn.net/doc/6id91fqsmz?spm=1055.2569.3001.10343)
阅读全文
相关推荐




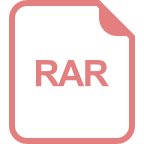
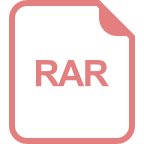
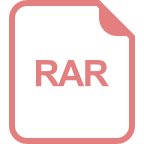


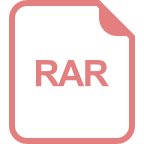







