使用jupyter解决BP神经网络识别鸢尾花数据集
时间: 2024-11-17 12:29:55 浏览: 43
在Jupyter Notebook中使用BP(Backpropagation,反向传播)神经网络对鸢尾花数据集进行分类,你需要做以下几个步骤:
1. **导入所需的库**:
```python
import numpy as np
from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.preprocessing import StandardScaler
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Dense
```
2. **加载并预处理数据**:
```python
iris = load_iris()
X = iris.data
y = iris.target
# 分割训练集和测试集
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42)
# 数据标准化
scaler = StandardScaler()
X_train = scaler.fit_transform(X_train)
X_test = scaler.transform(X_test)
```
3. **构建BP神经网络模型**:
```python
model = Sequential([
Dense(16, input_shape=(4,), activation='relu'), # 输入层和隐藏层
Dense(3, activation='softmax') # 输出层,3个节点对应3个类别
])
model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
```
4. **训练模型**:
```python
history = model.fit(X_train, y_train, epochs=100, validation_data=(X_test, y_test))
```
5. **评估模型性能**:
```python
_, accuracy = model.evaluate(X_test, y_test)
print(f"Accuracy on test set: {accuracy*100:.2f}%")
```
6. **可视化学习过程**(如果有需要):
```python
import matplotlib.pyplot as plt
plt.plot(history.history['accuracy'], label='Training Accuracy')
plt.plot(history.history['val_accuracy'], label='Validation Accuracy')
plt.xlabel('Epochs')
plt.ylabel('Accuracy')
plt.legend()
plt.show()
```
完成以上步骤后,你就有了一个使用BP神经网络在鸢尾花数据集上进行分类的基本流程。
阅读全文
相关推荐
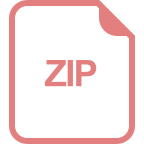
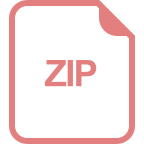
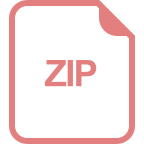
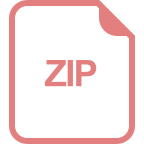
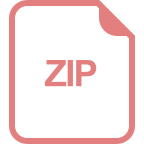
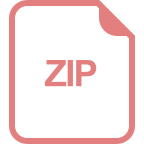
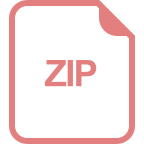
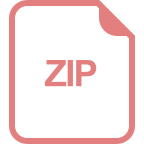
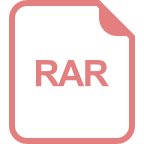
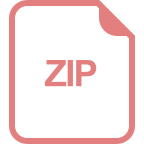
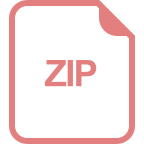
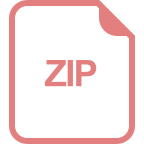
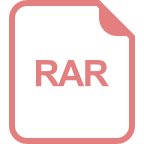
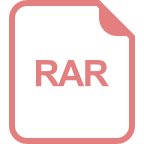
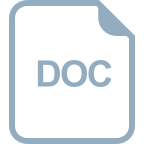
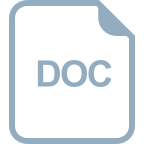
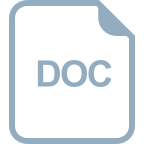