vue3实现列表的搜索与排序
时间: 2024-11-23 17:38:58 浏览: 6
Vue3实现列表的搜索与排序通常会结合Vuex管理状态、模板组件以及自定义事件。下面是一个简单的步骤说明:
1. **创建状态管理**:使用Vuex来存储列表数据,包括搜索关键字和排序规则。例如,你可以有一个`listState`对象,包含`data`, `searchKey`, 和 `sortField`等属性。
```javascript
import { createStore } from 'vuex';
const store = createStore({
state: {
listData: [], // 列表初始数据
searchKey: '',
sortField: '', // 排序字段默认为空
},
mutations: {
// 更新列表数据的相关方法
},
actions: {
fetchList({ commit }) {
// 获取列表数据的API调用
},
setSearchKey({ commit }, key) {
commit('setSearchKey', key);
},
setSortField({ commit }, field) {
commit('setSortField', field);
}
},
});
```
2. **响应式监听**:在组件中,通过`mapState`和`mapActions`从store获取并更新数据。
```html
<template>
<div>
<input v-model="searchKey" @input="onSearchChange" />
<select v-model="sortField" @change="onSortChange">
<!-- 提供排序选项 -->
</select>
<ul>
<li v-for="(item, index) in filteredItems" :key="index">{{ item }}</li>
</ul>
</div>
</template>
<script setup>
import { useStore } from 'vue.Store';
const store = useStore();
...
</script>
```
3. **过滤和排序函数**:在`setup`里,创建`filteredItems` computed property,用于实时过滤和排序列表。
```javascript
const filteredItems = computed(() => {
return store.state.listData.filter(item =>
item.name.includes(searchKey)
).sort((a, b) => {
if (sortField === 'name') {
return a.name.localeCompare(b.name);
} else if (sortField === 'date') {
// 对日期进行比较
}
return 0;
});
});
// 事件处理器
function onSearchChange(e) {
store.commit('setSearchKey', e.target.value);
}
function onSortChange() {
store.commit('setSortField', this.sortField); // 这里的this.sortField取自<select>的选择值
}
```
4. **在store中处理请求和数据更改**:当你触发搜索或排序操作时,`actions`中的相应方法会被调用,更新store内的状态,并通知所有依赖于该状态的组件。
阅读全文
相关推荐
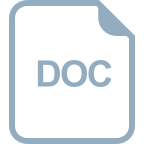
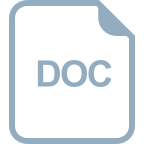
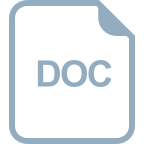

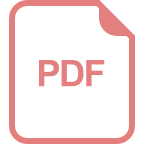
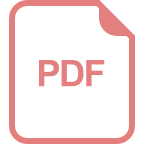
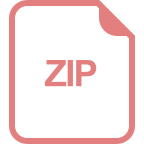
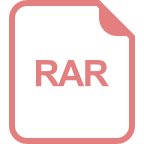
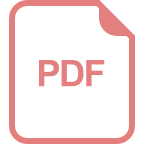
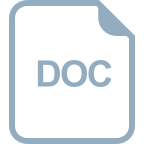
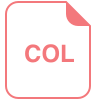
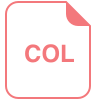




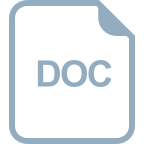
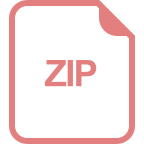