用c++语言详细地写一个使用模拟算法解决0-1背包问题程序
时间: 2024-12-10 21:36:03 浏览: 29
在C++中,使用模拟退火(Simulated Annealing)算法来解决0-1背包问题并不是常见的做法,因为遗传算法更适合此类优化问题。然而,如果你确实想要演示一种非标准但可以用于启发式搜索的模拟退火策略,你可以参考以下简化版的示例,但这并不适用于实际的0-1背包问题,因为它通常使用动态规划[^2]。请注意,这里我们将展示一个基本框架,而不是完整的解决方案:
```cpp
#include <iostream>
#include <vector>
#include <random>
// 假设我们有一个物品数组items和一个容量限制capacity
std::vector<int> items;
int capacity;
// 模拟退火函数
bool accept(int currentFitness, int newFitness, double temperature, double acceptanceProbability) {
if (newFitness > currentFitness) return true; // 如果新解更好,总是接受
else return std::exp(-(newFitness - currentFitness) / temperature) >= random_generator(); // 接受概率基于当前温度
}
void simulatedAnnealing() {
int currentSolutionFitness = calculateFitness(items); // 初始化初始解的适应度
int bestSolutionFitness = currentSolutionFitness;
double initialTemperature = 1000.0;
double coolingRate = 0.99;
while (temperature > 0.01) {
// 生成随机邻居解
std::vector<int> neighborItems(items);
// 进行随机交换或其他变异操作
int neighborFitness = calculateFitness(neighborItems);
bool accepted = accept(currentSolutionFitness, neighborFitness, temperature, 1.0);
if (accepted || neighborFitness > bestSolutionFitness) {
items = neighborItems; // 更新当前解
bestSolutionFitness = neighborFitness;
}
temperature *= coolingRate; // 冷却过程
}
std::cout << "Best solution found: " << bestSolutionFitness << std::endl;
}
// 虚拟的计算适应度函数,需根据具体问题定义
int calculateFitness(std::vector<int>& items) { /* ... */ }
int main() {
// 初始化物品和容量...
simulatedAnnealing();
return 0;
}
```
阅读全文
相关推荐







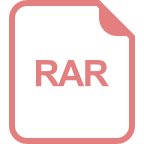
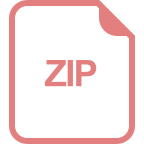






