生成十个入门级简单Python小程序(循环以前)
时间: 2024-09-23 12:01:27 浏览: 28
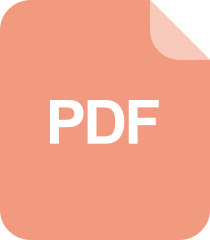
20行python代码的入门级小游戏的详解
当然可以,以下是十个适合初学者的简单Python小程序示例,它们主要涉及到基础语法、条件语句和循环结构:
1. **Hello World**:
```python
print("Hello, Python!")
```
2. **数数游戏**:
```python
for i in range(1, 11):
print(i)
```
3. **打印斐波那契数列**:
```python
a, b = 0, 1
while a < 10:
print(a)
a, b = b, a + b
```
4. **猜数字游戏**:
```python
import random
number = random.randint(1, 10)
guess = None
while guess != number:
guess = int(input("Guess a number between 1 and 10: "))
print("You got it!")
```
5. **计算列表平均值**:
```python
numbers = [1, 2, 3, 4, 5]
total = sum(numbers)
average = total / len(numbers)
print("Average:", average)
```
6. **字符串翻转**:
```python
input_string = input("Enter a string: ")
reversed_string = input_string[::-1]
print("Reversed String:", reversed_string)
```
7. **判断闰年**:
```python
year = int(input("Enter a year: "))
if (year % 4 == 0 and year % 100 != 0) or year % 400 == 0:
print(year, "is a leap year.")
else:
print(year, "is not a leap year.")
```
8. **计数器程序**:
```python
word = input("Enter a word: ")
word_count = word.count("a")
print("Number of 'a's:", word_count)
```
9. **简单的石头-剪刀-布游戏**:
```python
choices = ["rock", "paper", "scissors"]
player = input("Your choice: ").lower()
computer = random.choice(choices)
print(f"Computer chose {computer}. You win {player} vs {computer}?")
```
10. **打印九九乘法表**:
```python
for i in range(1, 10):
for j in range(1, i+1):
print(f"{j}*{i}={i*j}", end="\t")
print()
```
阅读全文
相关推荐
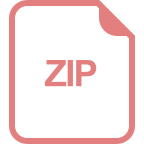
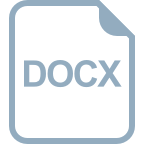

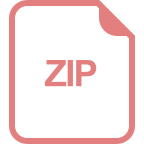
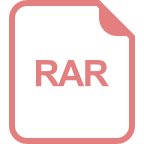
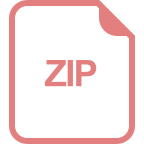
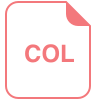
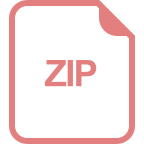
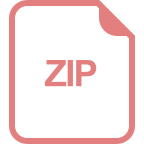
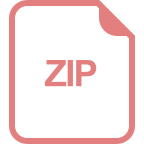
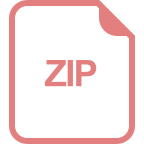
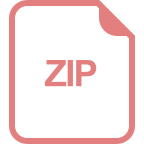
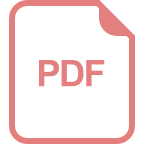
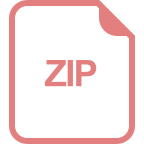
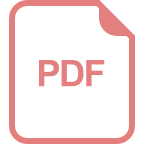
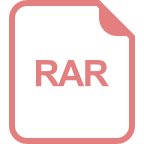
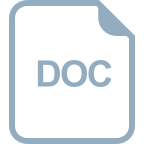