scala 使用slick框架处理多个left join查询时如何根据某个字段去重mysql中的数据
时间: 2024-04-13 13:28:28 浏览: 114
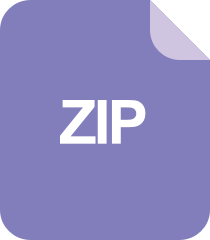
基于Scala的Apache Spark大数据处理框架设计源码
在使用 Slick 框架处理多个 left join 查询时,要根据某个字段去重 MySQL 中的数据,可以使用 Slick 提供的 `distinct` 方法来实现。以下是一个示例代码:
```scala
import slick.jdbc.MySQLProfile.api._
// 定义表的映射
class TableA(tag: Tag) extends Table[(Int, String)](tag, "table_a") {
def id = column[Int]("id", O.PrimaryKey)
def name = column[String]("name")
def * = (id, name)
}
class TableB(tag: Tag) extends Table[(Int, Int)](tag, "table_b") {
def id = column[Int]("id", O.PrimaryKey)
def tableAId = column[Int]("table_a_id")
def * = (id, tableAId)
// 外键关联
def tableAFk = foreignKey("table_a_fk", tableAId, TableA)(_.id)
}
// 创建表对象
val tableA = TableQuery[TableA]
val tableB = TableQuery[TableB]
// 查询并去重
val query = for {
(a, b) <- tableA joinLeft tableB on (_.id === _.tableAId)
} yield a
val distinctQuery = query.distinct
// 执行查询
val db = Database.forConfig("mysql")
val result = db.run(distinctQuery.result)
// 处理查询结果
result.onComplete {
case Success(data) => println(data)
case Failure(ex) => println("An error occurred: " + ex.getMessage)
}
```
上述代码中,我们定义了两个表 `TableA` 和 `TableB`,然后进行 left join 查询,并使用 `distinct` 方法对查询结果进行去重。最后,通过 `db.run` 方法执行查询,并处理查询结果。
请根据你的实际需求修改表的映射及查询逻辑。
阅读全文
相关推荐
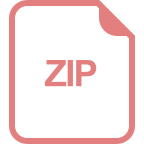
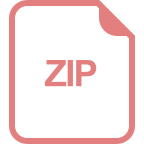


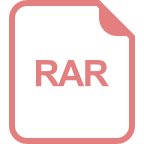
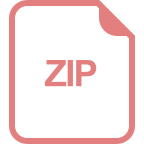
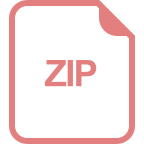
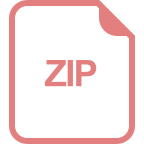
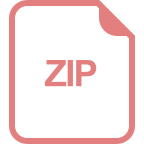
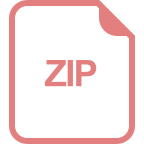
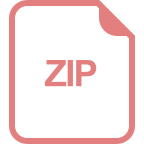
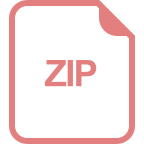
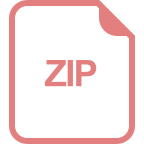
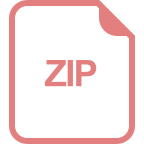
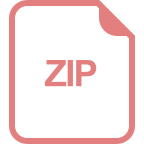
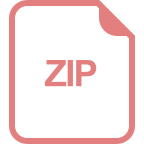
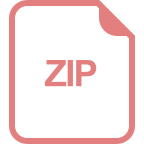