c# chrome tabcontrol
时间: 2025-01-03 10:37:26 浏览: 6
### 创建类似 Chrome 浏览器标签页效果的 TabControl
#### 在 C# WinForms 中实现
为了在WinForms应用程序中创建具有Chrome风格的`TabControl`,可以通过自定义样式和行为来增强默认的`TabControl`。下面是一个简单的例子:
```csharp
using System;
using System.Drawing;
using System.Windows.Forms;
public class CustomTabControl : TabControl {
protected override void OnPaint(PaintEventArgs e) {
Rectangle bounds = DisplayRectangle;
using (SolidBrush brush = new SolidBrush(Color.White)) {
e.Graphics.FillRectangle(brush, bounds);
}
int count = base.TabCount;
for(int i=0; i<count;i++) {
DrawTab(e.Graphics,i,bounds.Top);
}
}
private void DrawTab(Graphics g,int index,int top){
var tabRect = GetTabRect(index);
Color backColor = SelectedIndex == index ? Color.LightBlue : Color.Gainsboro;
using(SolidBrush brush=new SolidBrush(backColor)){
g.FillRectangle(brush,tabRect.X-1,top,tabRect.Width+2,tabRect.Height);
}
TextRenderer.DrawText(g,
Tabs[index].Text,
Font,
tabRect.Location,
ForeColor,
TextFormatFlags.HorizontalCenter | TextFormatFlags.VerticalCenter);
}
}
```
此代码片段展示了如何通过重写`OnPaint`方法来自定义`TabControl`外观[^1]。
#### 在 WPF 中实现
对于WPF应用而言,利用其强大的数据绑定能力和样式系统能够更方便地构建复杂的UI组件。这里提供一种基于模板的方式定制`TabControl`:
```xml
<TabControl>
<TabControl.Resources>
<!-- 定义ItemContainerStyle -->
<Style TargetType="TabItem">
<Setter Property="HeaderTemplate">
<Setter.Value>
<DataTemplate>
<StackPanel Orientation="Horizontal">
<TextBlock Text="{Binding}" Margin="5"/>
<Button Content="×" CommandParameter="{Binding RelativeSource={RelativeSource AncestorType=TabItem}}"
Click="CloseTab_Click"
Width="16" Height="16"/>
</StackPanel>
</DataTemplate>
</Setter.Value>
</Setter>
</Style>
<!-- 设置整体布局 -->
<Style TargetType="TabControl">
<Setter Property="Background" Value="#FFF"/>
<Setter Property="BorderThickness" Value="0"/>
<Setter Property="Padding" Value="4"/>
<Setter Property="Margin" Value="8"/>
<Setter Property="ItemsPanel">
<Setter.Value>
<ItemsPanelTemplate>
<WrapPanel IsItemsHost="True" />
</ItemsPanelTemplate>
</Setter.Value>
</Setter>
</Style>
</TabControl.Resources>
</TabControl>
```
上述XAML代码实现了带关闭按钮(`×`)的可点击标签项,并调整了整个控件的基础属性以匹配现代浏览器的设计理念。
此外,在后台逻辑处理方面,当用户尝试关闭某个选项卡时,可以在事件处理器里移除对应的`TabItem`对象:
```csharp
private void CloseTab_Click(object sender,RoutedEventArgs e){
Button button=(Button)sender;
TabItem item=((FrameworkElement)button).DataContext as TabItem;
((TabControl)item.Parent).Items.Remove(item);
}
```
这种做法不仅限于模仿特定软件界面;更重要的是学习到了如何灵活运用平台特性去创造满足需求的独特用户体验。
阅读全文
相关推荐






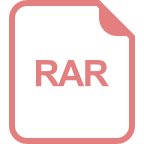
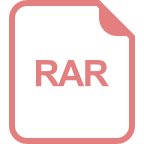
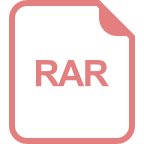
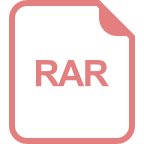




