javafx 文件选择填充
时间: 2025-01-06 12:46:04 浏览: 6
### JavaFX 中实现文件选择器并填充内容
在 JavaFX 应用程序中,可以利用 `FileChooser` 类来提供给用户一个图形化的接口用于选择文件。一旦选择了文件,则可以通过读取该文件的内容并将这些数据显示到界面上。
下面是一个简单的例子展示如何创建文件选择对话框以及加载选定文本文件中的数据至 `TextArea` 组件内:
```java
import javafx.application.Application;
import javafx.geometry.Insets;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.TextArea;
import javafx.scene.layout.VBox;
import javafx.stage.FileChooser;
import javafx.stage.Stage;
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class FileSelectorExample extends Application {
@Override
public void start(Stage primaryStage) {
Button btnSelectFile = new Button("选择文件");
TextArea textAreaContent = new TextArea();
textAreaContent.setPrefHeight(300);
VBox layout = new VBox(10, btnSelectFile, textAreaContent);
layout.setPadding(new Insets(20));
Scene scene = new Scene(layout, 400, 400);
// 设置按钮点击事件处理逻辑
btnSelectFile.setOnAction(event -> {
FileChooser fileChooser = new FileChooser(); // 创建文件选择器实例
// 可选配置:设置初始目录、过滤条件等...
// 显示打开文件对话框,并获取所选文件路径
var selectedFile = fileChooser.showOpenDialog(primaryStage);
if (selectedFile != null) { // 如果有文件被成功选取
try (BufferedReader br = new BufferedReader(new FileReader(selectedFile))) {
StringBuilder sb = new StringBuilder();
String line;
while ((line = br.readLine()) != null) {
sb.append(line).append("\n"); // 将每一行追加到字符串构建器里
}
textAreaContent.setText(sb.toString()); // 把整个文件内容显示出来
} catch (IOException ex) {
System.err.println(ex.getMessage());
}
}
});
primaryStage.setTitle("JavaFX 文件选择器示例");
primaryStage.setScene(scene);
primaryStage.show();
}
}
```
这段代码展示了如何使用 `javafx.stage.FileChooser` 来弹出文件选择窗口让用户挑选文件[^1]。接着通过标准 IO 流的方式逐行读入文件内容,并最终呈现在 GUI 上的一个多行文本区域 (`TextArea`) 内[^2]。
对于不同类型的文件(比如图片),则可能需要采用不同的方式去解析和呈现其内容;而对于某些特定格式的数据文件来说,还可以考虑引入第三方库来进行更加专业的解析工作。
阅读全文
相关推荐









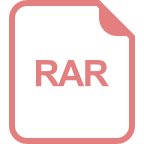







