python wxwidgets 串口通信GUI 完整代码
时间: 2024-09-21 14:05:10 浏览: 51
Python 中使用 `wxWidgets` 创建一个串口通信的 GUI,需要结合 `pySerial` 库来实现串口交互。下面是一个简单的示例代码,展示了一个窗口,用户可以选择串口号和波特率,然后可以发送和接收串口数据:
```python
import wx
from pyserial import Serial
class MyFrame(wx.Frame):
def __init__(self, parent=None):
super().__init__(parent, title="Python Serial GUI", size=(400, 300))
self.serial = None
# 初始化串口选择面板
serial_panel = wx.Panel(self)
serial_box_sizer = wx.BoxSizer(wx.VERTICAL)
self.combo_port = wx.ComboBox(serial_panel, choices=Serial().list_ports(), style=wx.CB_READONLY)
self.text_ctrl_baudrate = wx.TextCtrl(serial_panel, value="9600")
send_button = wx.Button(serial_panel, label="Send")
receive_button = wx.Button(serial_panel, label="Receive")
serial_box_sizer.Add(self.combo_port, proportion=1, flag=wx.EXPAND)
serial_box_sizer.Add(wx.StaticText(serial_panel, label="Baud Rate"), flag=wx.LEFT, border=5)
serial_box_sizer.Add(self.text_ctrl_baudrate, flag=wx.EXPAND)
serial_box_sizer.Add(send_button, flag=wx.ALL, border=5)
serial_box_sizer.Add(receive_button, flag=wx.ALL, border=5)
serial_panel.SetSizer(serial_box_sizer)
# 发送按钮事件处理器
def on_send_click(event):
baud_rate = int(self.text_ctrl_baudrate.GetValue())
try:
self.connect_serial(baud_rate)
self.send_data("Hello from GUI!")
except Exception as e:
wx.MessageBox(str(e), "Error", wx.OK | wx.ICON_ERROR)
send_button.Bind(wx.EVT_BUTTON, on_send_click)
# 接收数据处理
def on_receive_click(event):
try:
data = self.receive_data()
wx.MessageBox(f"Received data: {data}", "Message", wx.OK)
except Exception as e:
wx.MessageBox(str(e), "Error", wx.OK | wx.ICON_ERROR)
receive_button.Bind(wx.EVT_BUTTON, on_receive_click)
def connect_serial(self, baud_rate):
self.serial = Serial(port=self.combo_port.GetStringSelection(), baudrate=baud_rate)
self.serial.timeout = 1 # 设置超时时间,防止阻塞
def send_data(self, data):
if self.serial:
self.serial.write(data.encode('utf-8'))
print(f"Sent: {data}")
def receive_data(self):
if self.serial:
response = self.serial.read_until(b'\r\n') # 假设数据是以换行符分隔
return response.decode().strip()
if __name__ == "__main__":
app = wx.App()
frame = MyFrame()
frame.Show(True)
app.MainLoop()
```
这个例子中,我们创建了两个按钮,当点击“Send”按钮时会连接到选定的串口并发送数据,点击“Receive”按钮则尝试从串口读取数据。注意实际使用时需要对异常情况进行处理。
阅读全文
相关推荐
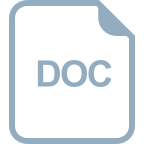
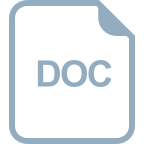
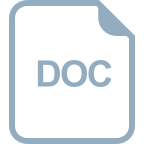

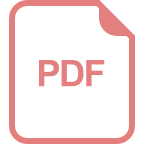
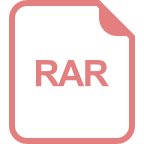
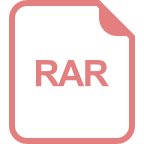
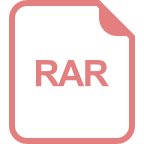
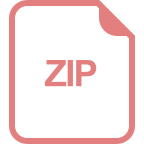
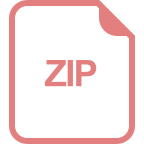
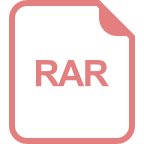
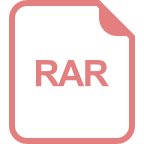
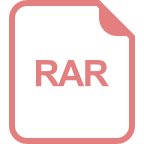
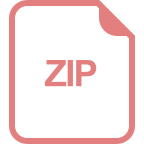
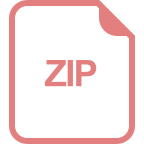
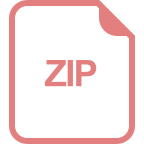
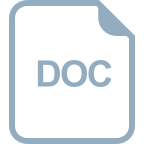
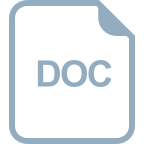
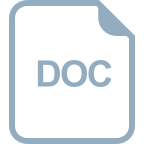