写一个简答的手部追踪代码
时间: 2024-09-06 17:07:22 浏览: 45
手部追踪通常涉及到计算机视觉中的深度传感器(如Microsoft Kinect或Intel RealSense)或者使用基于机器学习的手势识别技术。这里我将提供一个基本的Python代码片段,使用OpenCV和Dlib库进行简单的手势检测,这并不完全等同于精确的手部跟踪,但它可以帮助你在图像上定位手部。
首先,确保安装了必要的库:
```bash
pip install opencv-python numpy dlib
```
然后,你可以尝试下面的代码:
```python
import cv2
from imutils import face_utils
import dlib
# 加载预训练的人脸和手部关键点模型
detector = dlib.get_frontal_face_detector()
predictor = dlib.shape_predictor('shape_predictor_68_face_landmarks.dat')
handlandmarkDetector = dlib.shape_predictor('hand_landmarks_model.dat')
while True:
# 读取摄像头画面
cap = cv2.VideoCapture(0) # 0代表默认摄像头
ret, frame = cap.read()
# 转换为灰度图像以便更容易检测
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
# 检测人脸
faces = detector(gray)
for face in faces:
# 获取面部特征
landmarks = predictor(gray, face)
# 为了更好地显示手部,我们只关心左手和右手
left_hands = handlandmarkDetector(gray, face.left() + face.width() * 0.1) # 假设手位于脸部右侧
right_hands = handlandmarkDetector(gray, face.right() - face.width() * 0.1) # 假设手位于脸部左侧
# 如果有手部数据可用
if left_hands or right_hands:
# 根据需要处理手部的关键点数据,例如绘制手指轮廓
for i in range(1, 21): # 手部有21个关键点
if left_hands:
(x, y) = left_hands.part(i).position
else:
(x, y) = right_hands.part(i).position
cv2.circle(frame, (x, y), 3, (0, 255, 0), -1)
# 显示结果
cv2.imshow('Hand Tracking', frame)
# 按 'q' 键退出
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# 释放资源
cap.release()
cv2.destroyAllWindows()
```
这个代码简单地在摄像头视频上标出检测到的手部关键点。注意,这是一个基础示例,实际的手部跟踪可能需要更复杂的算法,并结合深度信息来获得更准确的结果。
阅读全文
相关推荐
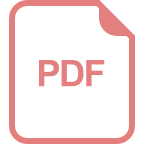
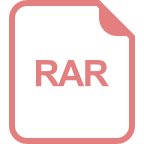
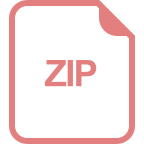
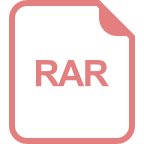
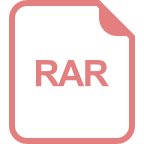
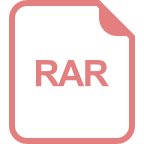
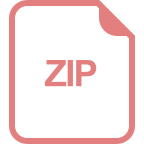
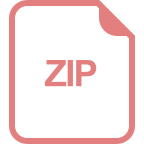
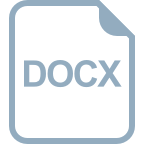
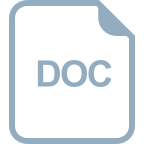