多目标规划遗传算法代码
时间: 2024-04-08 18:26:51 浏览: 100
多目标规划遗传算法是一种用于解决多目标优化问题的进化算法。下面是一个简单的多目标规划遗传算法的代码示例:
```python
import random
# 定义目标函数
def objective_function(x):
return [x[0]**2, (x[0]-2)**2]
# 定义个体类
class Individual:
def __init__(self, chromosome_length, lower_bound, upper_bound):
self.chromosome = [random.uniform(lower_bound, upper_bound) for _ in range(chromosome_length)]
self.fitness = None
def evaluate(self):
self.fitness = objective_function(self.chromosome)
# 定义种群类
class Population:
def __init__(self, population_size, chromosome_length, lower_bound, upper_bound):
self.population = [Individual(chromosome_length, lower_bound, upper_bound) for _ in range(population_size)]
def evaluate(self):
for individual in self.population:
individual.evaluate()
def select_parents(self, num_parents):
parents = []
sorted_population = sorted(self.population, key=lambda x: x.fitness)
for i in range(num_parents):
parents.append(sorted_population[i])
return parents
def crossover(self, parents, offspring_size):
offspring = []
for _ in range(offspring_size):
parent1 = random.choice(parents)
parent2 = random.choice(parents)
child = Individual(len(parent1.chromosome), min(parent1.chromosome), max(parent1.chromosome))
for i in range(len(parent1.chromosome)):
if random.random() < 0.5:
child.chromosome[i] = parent1.chromosome[i]
else:
child.chromosome[i] = parent2.chromosome[i]
offspring.append(child)
return offspring
def mutate(self, offspring, mutation_rate):
for individual in offspring:
for i in range(len(individual.chromosome)):
if random.random() < mutation_rate:
individual.chromosome[i] = random.uniform(min(individual.chromosome), max(individual.chromosome))
# 定义多目标规划遗传算法类
class MOGA:
def __init__(self, population_size, chromosome_length, lower_bound, upper_bound, num_generations, num_parents, offspring_size, mutation_rate):
self.population = Population(population_size, chromosome_length, lower_bound, upper_bound)
self.num_generations = num_generations
self.num_parents = num_parents
self.offspring_size = offspring_size
self.mutation_rate = mutation_rate
def run(self):
for _ in range(self.num_generations):
self.population.evaluate()
parents = self.population.select_parents(self.num_parents)
offspring = self.population.crossover(parents, self.offspring_size)
self.population.mutate(offspring, self.mutation_rate)
self.population.population = parents + offspring
self.population.evaluate()
best_individual = min(self.population.population, key=lambda x: x.fitness)
return best_individual.chromosome
# 使用示例
population_size = 50
chromosome_length = 1
lower_bound = -5
upper_bound = 5
num_generations = 100
num_parents = 20
offspring_size = 30
mutation_rate = 0.1
moga = MOGA(population_size, chromosome_length, lower_bound, upper_bound, num_generations, num_parents, offspring_size, mutation_rate)
best_solution = moga.run()
print("Best solution:", best_solution)
```
这段代码实现了一个简单的多目标规划遗传算法,其中包括个体类、种群类和多目标规划遗传算法类。通过调整参数,可以适应不同的问题和需求。
阅读全文
相关推荐
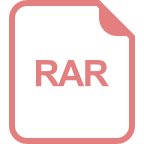
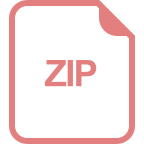
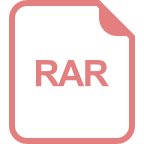
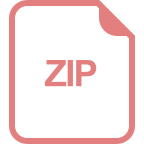
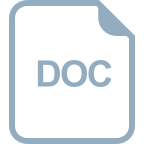
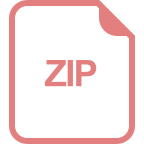
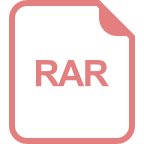
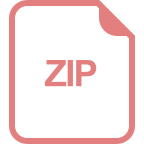
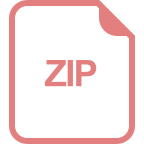
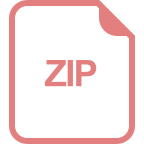
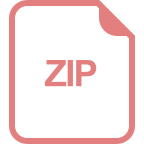
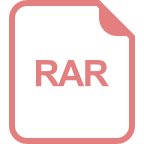
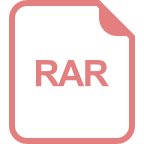
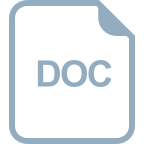
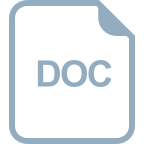

