有 � n个人在一艘海上航行的船上,现在遇到了灾难,船上只能承载一个人了,现在人们想用投票的方式决定谁留在船上,投票的方式如下: � n个人按编号 1 ∼ � 1∼n站成一个环形,从第一个人开始从 1 1报数,报到 � m的人踢出队列,从下一个人开始重新从 1 1报数。最后剩下的留在船上。 现在问这场生存之战最后留在船上的编号是多少。
时间: 2024-04-17 10:25:06 浏览: 168
这个问题可以使用循环链表来解决,每个节点表示一个人,通过不断删除节点来模拟报数过程。
下面是使用 C 语言编写的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构体
typedef struct Node {
int number;
struct Node* next;
} Node;
// 创建循环链表
Node* createCircularLinkedList(int n) {
Node* head = NULL;
Node* prev = NULL;
for (int i = 1; i <= n; i++) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->number = i;
newNode->next = NULL;
if (head == NULL) {
head = newNode;
} else {
prev->next = newNode;
}
prev = newNode;
}
prev->next = head; // 将链表首尾相连形成循环
return head;
}
// 删除指定节点,并返回下一个节点
Node* deleteNode(Node* node) {
Node* nextNode = node->next;
node->next = nextNode->next;
free(nextNode);
return node->next;
}
// 寻找最后留在船上的编号
int findLastSurvivor(int n, int m) {
Node* head = createCircularLinkedList(n);
Node* current = head;
// 找到要删除的节点的前一个节点
for (int i = 0; i < n - 1; i++) {
for (int j = 0; j < m - 2; j++) {
current = current->next;
}
current = deleteNode(current);
}
int lastSurvivor = current->number;
free(current);
return lastSurvivor;
}
int main() {
int n, m;
printf("请输入人数n:");
scanf("%d", &n);
printf("请输入报数m:");
scanf("%d", &m);
int lastSurvivor = findLastSurvivor(n, m);
printf("最后留在船上的编号是:%d\n", lastSurvivor);
return 0;
}
```
用户需要输入人数 n 和报数 m。程序会计算出最后留在船上的编号,并输出结果。
阅读全文
相关推荐
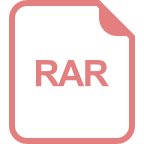

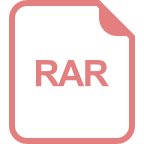







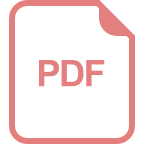


