Python写一个识别照片里面的内容,照片都在一个文件夹里面,有预览按键选择文件,然后识别照片里面的内容识别学习记录,有50多张照片,识别结束后显示有多少张照片的里面有这个字,有多少张没有,用qt5
时间: 2024-09-09 21:13:02 浏览: 55
在Qt5中,你可以创建一个简单的图形用户界面(GUI)来实现这个功能。这里是一个基本的框架,我们将使用Pillow库进行图像预处理,以及pytesseract进行光学字符识别(OCR),同时利用QFileDialog选择文件和QLabel显示结果。注意,由于篇幅限制,下面的代码片段仅展示了核心部分:
```python
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QWidget, QPushButton, QLabel, QVBoxLayout, QFileDialog
from PIL import Image
from pytesseract import image_to_string
import os
class PhotoRecognizer(QMainWindow):
def __init__(self):
super().__init__()
self.init_ui()
def init_ui(self):
self.setWindowTitle("照片识别")
main_widget = QWidget()
layout = QVBoxLayout(main_widget)
# 预览按钮
self.preview_button = QPushButton("浏览图片", self)
self.preview_button.clicked.connect(self.select_image)
layout.addWidget(self.preview_button)
# 结果展示
self.result_label = QLabel("", self)
layout.addWidget(self.result_label)
self.setCentralWidget(main_widget)
def select_image(self):
options = QFileDialog.Options()
options |= QFileDialog.DontUseNativeDialog
file_path, _ = QFileDialog.getOpenFileName(self, "选取图片", "", "All Files (*);;Image Files (*.png *.jpg)", options=options)
if file_path:
self.display_result(file_path)
def display_result(self, file_path):
try:
with Image.open(file_path) as img:
text = image_to_string(img)
if "你要找的字" in text:
result = f"{file_path} - 找到了"
else:
result = f"{file_path} - 没找到"
self.result_label.setText(result)
except Exception as e:
self.result_label.setText(f"无法识别 {file_path}: {str(e)}")
if __name__ == "__main__":
app = QApplication(sys.argv)
window = PhotoRecognizer()
window.show()
sys.exit(app.exec_())
```
此代码实现了基本的功能,点击“浏览图片”按钮后,会选择文件夹中的图片,识别内容并在结果显示区域显示。但请注意,实际项目中可能还需要处理更多细节,比如错误处理、进度反馈等,并且针对50多张照片的批量识别,你需要编写循环遍历文件夹的代码。
阅读全文
相关推荐
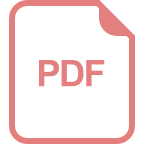
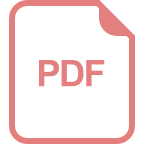
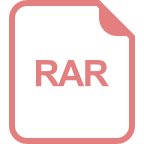
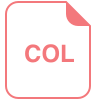
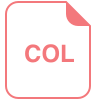
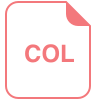
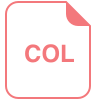
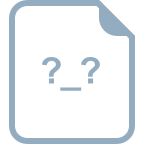
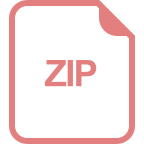
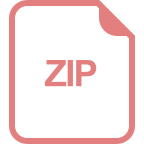
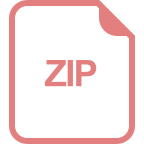
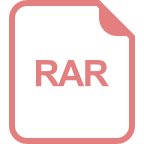
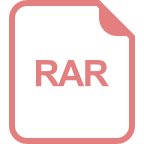
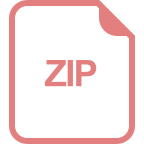
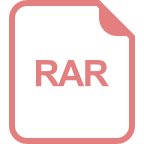
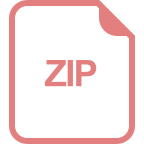
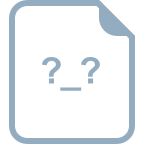
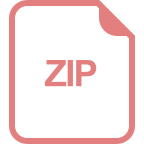