ts 对axios响应数据进行类型判断封装
时间: 2024-09-22 19:09:47 浏览: 48
在TypeScript (TS) 中,为了对 Axios 响应的数据进行类型安全的处理并封装,你可以创建一个自定义的函数或者模块,结合 Axios 的 `response` 属性和 TypeScript 的类型系统。以下是一个简单的例子:
```typescript
import axios from 'axios';
// 定义一个泛型类型的 Axios 请求拦截器
interface AxiosResponse<T> {
data: T;
}
// 封装 Axios 并添加类型判断
const api = axios.create({
baseURL: 'your_base_url',
responseType: 'json', // 设置默认返回JSON格式
});
api.interceptors.response.use(
(response: AxiosResponse<any>) => {
// 类型断言检查数据是否是我们期望的类型
if (typeof response.data === 'object' && !Array.isArray(response.data)) {
// 如果是对象并且不是数组,我们可以假设它是某种具体的对象类型T
const typedData: YourDataType = response.data as YourDataType;
// 进行进一步的操作或返回typedData
return { data: typedData };
} else {
return response; // 如果不符合预期,原样返回
}
},
(error) => {
// 处理错误...
return Promise.reject(error);
}
);
// 调用API示例
api.get('/your-endpoint').then((response) => {
// 现在你可以放心地使用typedData了,因为typescript已经帮我们进行了类型校验
console.log(response.data); // YourDataType
}).catch((error) => {
console.error(error);
});
阅读全文
相关推荐
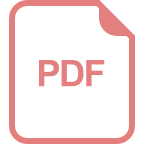
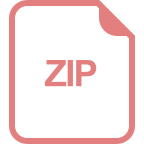
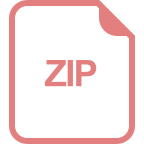
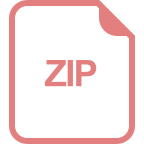


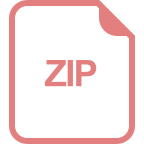
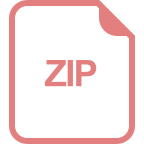
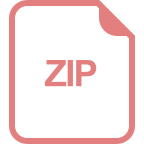
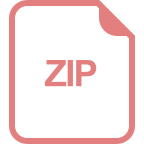
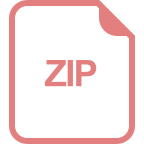
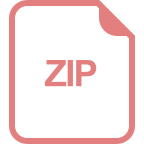
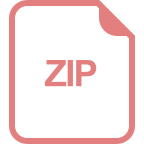
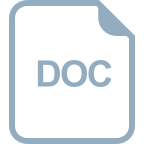


