c语言实现aes_ecb_cbc加密
时间: 2023-09-01 08:04:52 浏览: 137
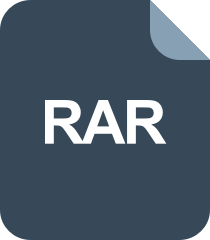
C语言实现AES_ECB加密

C语言中可以使用OpenSSL库来实现AES(ECB/CBC)加密算法。下面是一个简单的示例代码:
```c
#include <stdio.h>
#include <openssl/aes.h>
/* AES ECB加密 */
void aes_ecb_encrypt(const unsigned char *plaintext, const unsigned char *key, unsigned char *ciphertext) {
AES_KEY aes_key;
AES_set_encrypt_key(key, 128, &aes_key);
AES_ecb_encrypt(plaintext, ciphertext, &aes_key, AES_ENCRYPT);
}
/* AES CBC加密 */
void aes_cbc_encrypt(const unsigned char *plaintext, const unsigned char *key, const unsigned char *iv, unsigned char *ciphertext) {
AES_KEY aes_key;
AES_set_encrypt_key(key, 128, &aes_key);
AES_cbc_encrypt(plaintext, ciphertext, 128, &aes_key, iv, AES_ENCRYPT);
}
int main() {
unsigned char key[] = "0123456789ABCDEF"; // 16字节长度的密钥
unsigned char iv[] = "1234567890ABCDEF"; // 16字节长度的初始向量
unsigned char plaintext[] = "Hello, World!"; // 待加密的明文
unsigned char ecb_ciphertext[AES_BLOCK_SIZE];
unsigned char cbc_ciphertext[AES_BLOCK_SIZE];
aes_ecb_encrypt(plaintext, key, ecb_ciphertext);
aes_cbc_encrypt(plaintext, key, iv, cbc_ciphertext);
printf("ECB ciphertext: ");
for (int i = 0; i < AES_BLOCK_SIZE; i++) {
printf("%02x ", ecb_ciphertext[i]);
}
printf("\n");
printf("CBC ciphertext: ");
for (int i = 0; i < AES_BLOCK_SIZE; i++) {
printf("%02x ", cbc_ciphertext[i]);
}
printf("\n");
return 0;
}
```
上面的代码实现了AES-128算法的ECB和CBC模式的加密。通过`aes_ecb_encrypt`函数和`aes_cbc_encrypt`函数,可以将明文加密为对应的密文。其中,`key`和`iv`分别为密钥和初始向量,在实际应用中需要保证其安全性和随机性。
`main`函数中的示例对字符串"Hello, World!"进行了ECB和CBC加密,并打印出对应的密文。
阅读全文
相关推荐
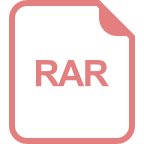
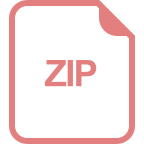
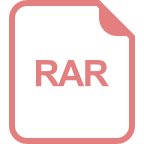
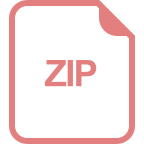
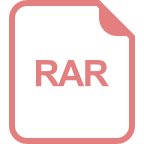
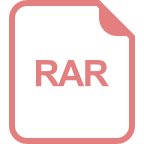
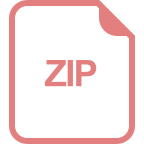
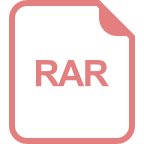
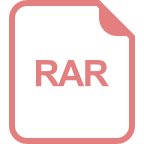
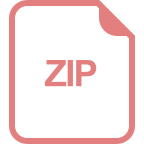
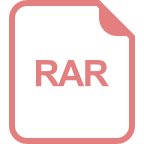
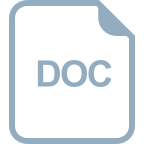
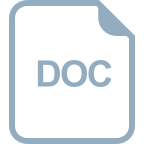
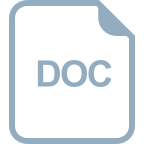
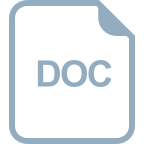
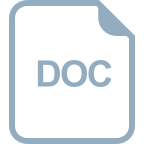