将 Datatype 类型定义为结构数组,做一个可以创建,查找,插入,删除的线性表 typedef struct{ int id; char name[20];//char *name; }Datatype;
时间: 2024-09-16 12:01:45 浏览: 42
Datatype 结构数组是一种数据结构,它将 id 和名字(字符数组形式)作为两个字段组合在一起,用于表示每个元素。在线性表的操作中,我们通常会定义一个数组来存储这种类型的元素,以便进行创建、查找、插入和删除操作。
1. 创建 (Insert):我们可以初始化一个空的 Datatype 数组,然后通过索引来添加新元素。例如:
```c
Datatype list[100]; // 假设数组大小为100
if (list_size < MAX_SIZE) {
list[list_size].id = new_id;
strcpy(list[list_size].name, "New Element");
list_size++;
}
```
2. 查找 (Search):通过指定 id 或 name 来搜索特定元素,例如:
```c
int find_element(Datatype *list, int id, int size){
for(int i = 0; i < size; i++){
if(list[i].id == id){
return i;
}
}
return -1; // 如果未找到则返回 -1
}
```
3. 插入 (Insertion):如果需要在已排序列表中插入,可能需要先对元素进行比较,然后调整其他元素的位置:
```c
void insert_sorted(Datatype *list, int index, Datatype item){
if(index > list_size) index = list_size;
memmove(&list[index+1], &list[index], sizeof(Datatype)*(list_size-index));
list[index] = item;
list_size++;
}
```
4. 删除 (Deletion):移除指定位置的元素并调整后续元素:
```c
void delete_element(Datatype *list, int index, int size){
if(index < list_size){
memmove(&list[index], &list[index+1], sizeof(Datatype)*(list_size-1-index));
list_size--;
}
}
```
阅读全文
相关推荐
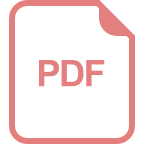
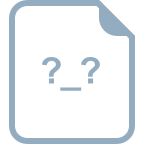



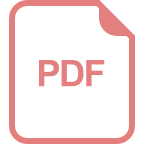
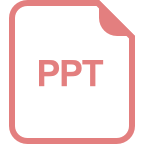
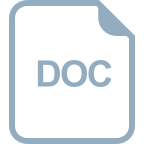
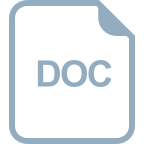
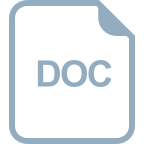
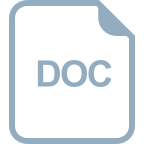






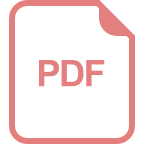