如何使用C语言构建一个整数类型的线性表,并将其元素34, 56, 20, 9, 15, 和5依次插入到该表中?
时间: 2024-11-03 09:13:05 浏览: 14
在C语言中,我们可以使用数组或链表来构建整数类型的线性表。以下是两种常见的做法:
**1. 数组实现**
```c
#include <stdio.h>
#define SIZE 6 // 线性表的最大元素个数
int linearList[SIZE]; // 声明整数数组
void insertIntoArray(int index, int value) {
// 验证索引范围
if (index >= 0 && index < SIZE) {
linearList[index] = value; // 插入元素
printf("Inserted %d at position %d.\n", value, index);
} else {
printf("Index out of bounds!\n");
}
}
int main() {
// 初始化数组,然后逐个插入元素
for (int i = 0; i <= 5; i++) { // 注意索引从0开始
insertIntoArray(i, 34 + i); // 依次插入34, 56, 20, 9, 15, 5
}
return 0;
}
```
**2. 链表实现(单向链表)**
```c
#include <stdio.h>
struct Node {
int data;
struct Node* next;
};
void insertAtEnd(struct Node** head, int value) {
if (*head == NULL) {
*head = (struct Node*)malloc(sizeof(struct Node));
(*head)->data = value;
(*head)->next = NULL;
} else {
struct Node* last = *head;
while (last->next != NULL) {
last = last->next;
}
last->next = (struct Node*)malloc(sizeof(struct Node));
last->next->data = value;
last->next->next = NULL;
}
printf("Inserted %d at the end.\n", value);
}
int main() {
struct Node* head = NULL;
for (int i = 0; i <= 5; i++) {
insertAtEnd(&head, 34 + i);
}
return 0;
}
```
在这两个示例中,我们已经按照顺序插入了给定的整数值。请注意,在数组实现中,元素会直接覆盖前面已存在的值;而在链表实现中,元素会被添加到链表的末尾。
阅读全文
相关推荐
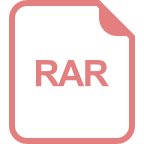
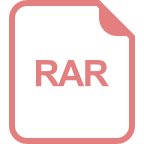
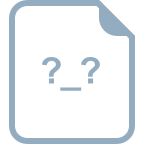
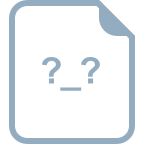
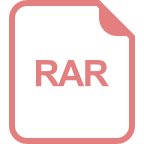
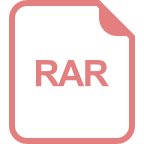
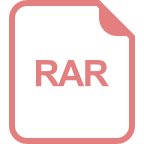
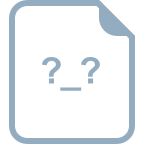
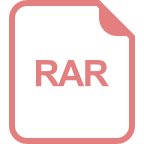
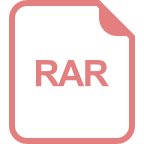
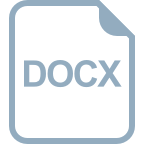
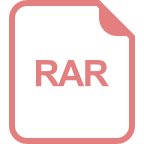
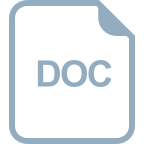