设计一个算法,求二叉树中值为x的结点的孩子结点。输出C++代码
时间: 2024-12-22 14:25:37 浏览: 8
为了在二叉树中寻找值为`x`的节点及其子节点,我们可以采用深度优先搜索(Depth First Search, DFS)策略。对于每个访问到的节点,如果它的值等于`x`,我们就记录它的左右子节点。这里是一个简单的C++代码实现:
```cpp
#include <iostream>
class TreeNode {
public:
int val;
TreeNode* left;
TreeNode* right;
TreeNode(int x) : val(x), left(nullptr), right(nullptr) {}
};
// 深度优先搜索,查找值为x的节点及其子节点
void findChildren(TreeNode* root, int x, TreeNode** child1, TreeNode** child2) {
if (!root) {
*child1 = *child2 = nullptr;
return;
}
if (root->val == x) {
*child1 = root->left;
*child2 = root->right;
} else if (root->val > x) {
findChildren(root->left, x, child1, child2);
} else {
findChildren(root->right, x, child1, child2);
}
}
// 打印节点
void printNode(TreeNode* node) {
if (node) {
std::cout << node->val;
if (node->left) printNode(node->left);
if (node->right) printNode(node->right);
}
}
int main() {
// 假设你已经构建了一个名为`root`的二叉树实例
int targetValue = 5;
TreeNode** child1 = &(*new TreeNode(0));
TreeNode** child2 = &(*new TreeNode(0));
findChildren(root, targetValue, child1, child2);
if (*child1) {
std::cout << "Left child of node with value " << targetValue << ":";
printNode(*child1);
}
if (*child2) {
std::cout << "\nRight child of node with value " << targetValue << ":";
printNode(*child2);
}
delete child1;
delete child2; // 释放动态分配的内存
return 0;
}
```
在这个代码中,`findChildren`函数遍历整个二叉树,直到找到值为`x`的节点。然后,它设置`child1`指向左子节点,`child2`指向右子节点。如果没有找到,对应的指针会被设置为`nullptr`。注意在结束时需要手动释放动态分配的内存。
阅读全文
相关推荐
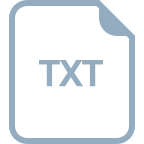
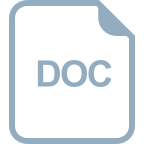
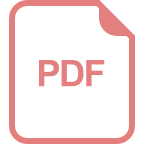
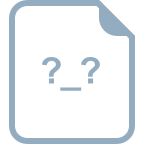
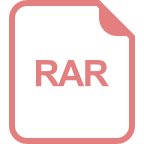
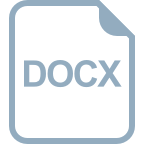
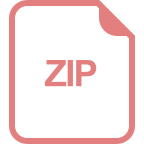
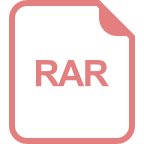
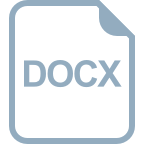
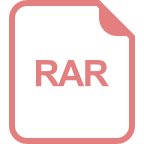
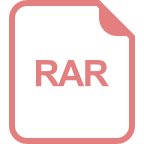