RSA_private_encrypt
时间: 2023-09-12 16:04:49 浏览: 359
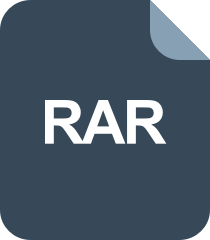
RSA加密
RSA_private_encrypt是一个RSA算法中的加密函数。它是使用私钥对数据进行加密的过程。RSA算法是一种非对称加密算法,使用两个密钥:公钥和私钥。公钥用于加密数据,私钥用于解密数据。
在使用RSA_private_encrypt函数时,你需要提供以下参数:
- data:要加密的数据
- data_len:数据的长度
- rsa_key:RSA私钥
函数会将输入的数据使用私钥进行加密,并返回加密后的结果。请注意,该函数仅适用于加密较小的数据块,因为RSA算法在处理大数据块时可能会很慢。
需要注意的是,我无法为你提供具体的代码示例或更多细节,因为我并不知道你要在哪种编程语言中使用该函数。如果你需要更多帮助,请参考相关编程语言的文档或搜索相关示例。
阅读全文
相关推荐
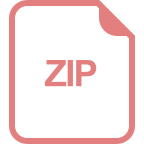
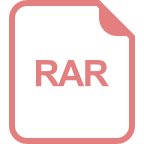

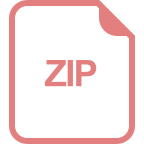
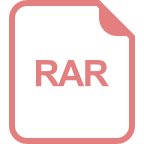
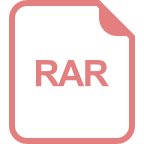
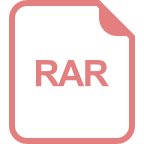
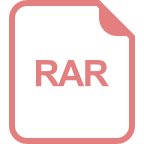
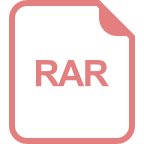
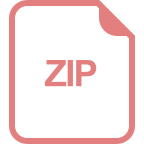
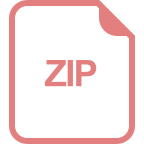
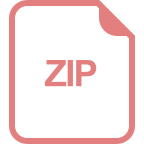
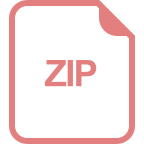
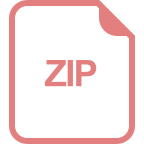
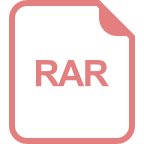
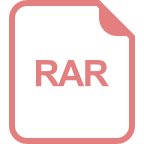
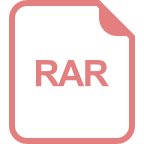
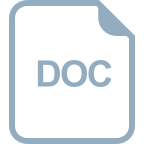