java代码:自己定义一个泛型类型的队列 MyQueue<T>,定义队列长度等成员变量,顶一个入队和出队等成员函数。写一个主类主函数,进行测试,建一个水果类Fruit类,定义若干个水果对象进行入队和出队操作。定义Student类,进行入队和出队测试。
时间: 2024-09-24 10:02:32 浏览: 51
在Java中,我们可以创建一个泛型类型的队列`MyQueue<T>`,这个队列可以存储任意类型的对象。首先,我们需要定义队列的成员变量,如容量、头元素和尾指针。这里假设我们有一个私有整数`queueSize`表示队列最大长度,两个节点引用`head`和`tail`分别表示队首和队尾。
```java
public class MyQueue<T> {
private int queueSize;
private int head; // 队首索引
private int tail; // 队尾索引
private T[] elements;
public MyQueue(int capacity) {
this.queueSize = capacity;
elements = (T[]) new Object[capacity];
head = -1;
tail = -1;
}
// 入队方法
public void enqueue(T item) {
if ((tail + 1) % queueSize == head) { // 当队满时,需要扩容
resize();
}
elements[++tail] = item;
if (head == -1) {
head = 0;
}
}
// 出队方法
public T dequeue() {
if (head == tail) { // 空队列异常
throw new IllegalStateException("Queue is empty");
}
T item = elements[head];
elements[head++] = null; // 移动头指针
return item;
}
// 扩容方法
private void resize() {
T[] oldElements = elements;
int newSize = oldElements.length * 2;
elements = (T[]) new Object[newSize];
System.arraycopy(oldElements, 0, elements, 0, tail + 1);
head = 0;
tail = tail < newSize - 1 ? tail : newSize - 1;
}
// 主函数示例
public static void main(String[] args) {
// 创建MyQueue实例,并作为演示如何操作
MyQueue<Fruit> fruitQueue = new MyQueue<>(5);
// 水果类Fruit的定义(省略)
class Fruit {}
// 测试水果队列
Fruit apple = new Fruit();
fruitQueue.enqueue(apple); // 进行入队操作
// 学生类Student的定义(省略)
class Student {}
// 测试学生队列
MyQueue<Student> studentQueue = new MyQueue<>(3);
Student student1 = new Student();
studentQueue.enqueue(student1); // 同样进行入队操作
}
}
```
在这个例子中,我们创建了两个队列,一个用于存储`Fruit`对象,另一个用于存储`Student`对象。注意,实际项目中你需要为`Fruit`和`Student`类提供完整的定义。
阅读全文
相关推荐
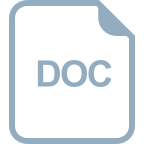
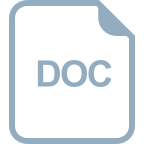
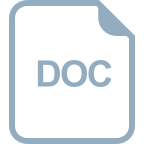

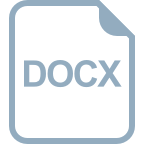
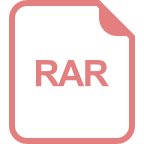
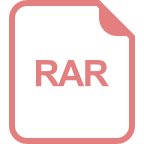
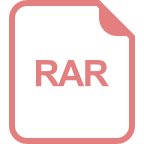
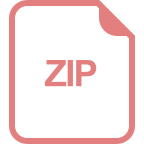
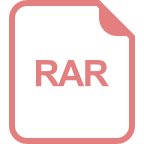
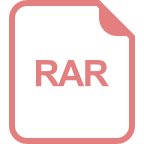
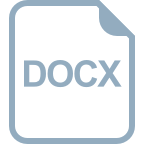
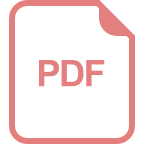
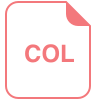
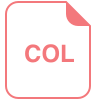
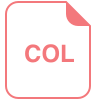
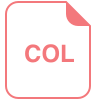
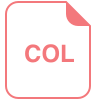
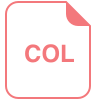