如何编写一个c语言程序来接收五个学生的成绩输入,然后计算并输出他们的总成绩和平均分?
时间: 2024-12-21 16:23:33 浏览: 18
编写一个C语言程序来接收五个学生的成绩并计算总成绩和平均分,可以按照以下步骤进行:
```c
#include <stdio.h> // 包含标准输入输出库
int main() {
int scores[5]; // 创建一个数组来存储五个学生的成绩
float total = 0; // 初始化总成绩为零
float average; // 初始化平均分为浮点数
// 循环接收五次输入
for (int i = 0; i < 5; i++) {
printf("请输入第 %d 位学生的成绩: ", i + 1);
scanf("%d", &scores[i]); // 输入整数并存储到数组中
total += scores[i]; // 累加每个学生的成绩
}
// 计算平均分
average = (float)total / 5; // 将总成绩转换为浮点数并除以学生人数
// 输出结果
printf("五名学生的总成绩是: %d\n", total);
printf("他们的平均成绩是: %.2f\n", average); // 保留两位小数
return 0;
}
```
在这个程序中,我们首先创建一个数组`scores`来存储成绩,然后用循环结构依次请求用户输入五次。每次输入都被添加到`total`上,最后计算出平均分并显示结果。
相关问题
编程求从键盘上输入一个学生的n门成绩,计算出该学生的总成绩和平均成绩。c语言
下面是一个简单的 C 语言程序,用于计算一个学生的 n 门成绩的总分和平均分。在程序中,我们使用了一个 for 循环来接收 n 门成绩,然后通过计算总分和平均分来输出结果。
```c
#include <stdio.h>
int main() {
int n;
float grade, total = 0, average;
printf("Enter the number of grades: ");
scanf("%d", &n);
for (int i = 1; i <= n; i++) {
printf("Enter grade %d: ", i);
scanf("%f", &grade);
total += grade;
}
average = total / n;
printf("Total grade: %.2f\n", total);
printf("Average grade: %.2f\n", average);
return 0;
}
```
在这个程序中,我们首先要求用户输入他们的成绩数量。然后,我们使用 for 循环从键盘上接收 n 个成绩,并将它们相加以计算总分。最后,我们计算平均分并输出结果。
请注意,我们使用 %.2f 格式说明符来输出浮点数,以显示两位小数。
怎么用C语言均计算多个人的总成绩和平均成绩
在C语言中,你可以创建一个函数来计算多个人的总成绩和平均成绩。首先,你需要定义一些结构体来存储每个人的信息,如姓名和成绩。然后定义一个数组来存储所有学生的数据。这里是一个简单的示例:
```c
#include <stdio.h>
#include <string.h>
// 定义学生结构体
typedef struct {
char name[50];
int score;
} Student;
// 计算总成绩和平均分的函数
void calculate_total_and_average(Student students[], int num_students) {
int total_score = 0;
float average;
// 遍历数组,累加总分
for (int i = 0; i < num_students; i++) {
total_score += students[i].score;
}
// 计算平均分并打印结果
average = (float)total_score / num_students;
printf("总成绩: %d\n", total_score);
printf("平均成绩: %.2f\n", average);
}
int main() {
// 假设有三个学生
Student students[] = {
{"张三", 85},
{"李四", 90},
{"王五", 95}
};
int num_students = sizeof(students) / sizeof(students[0]);
// 调用函数
calculate_total_and_average(students, num_students);
return 0;
}
```
在这个例子中,`calculate_total_and_average` 函数接收一个学生数组和人数作为输入,通过遍历数组计算总分,并将总分除以学生数得到平均分。
阅读全文
相关推荐








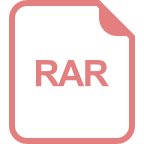
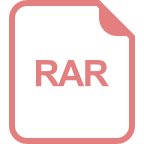



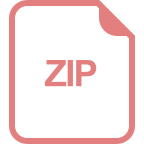


