Python爬虫图片的代码
时间: 2024-05-18 12:09:31 浏览: 107
当爬取网页中的图片时,我们需要用到Python中的requests库和BeautifulSoup库,下面是一个简单的Python爬虫图片的代码示例:
``` python
import requests
from bs4 import BeautifulSoup
# 定义要爬取的URL地址
url = "https://www.example.com"
# 发送请求并获取页面内容
response = requests.get(url)
html = response.content
# 使用BeautifulSoup库解析页面内容
soup = BeautifulSoup(html, 'html.parser')
img_tags = soup.find_all('img')
# 遍历所有的图片标签,获取图片链接并下载
for img in img_tags:
img_url = img.get('src')
# 使用requests库发送请求并下载图片
img_data = requests.get(img_url).content
with open('image_name.jpg', 'wb') as handler:
handler.write(img_data)
# 下载完成提示
print("所有图片已经下载完成!")
```
这是一个简单的爬虫示例,实际情况中还需要加入错误处理、多线程下载等功能,以提高代码的鲁棒性和效率。
相关问题
python爬虫万能代码-python网络爬虫源代码(可直接抓取图片)
下面是一个简单的 Python 网络爬虫源代码,可以抓取网页内容和图片:
```python
import requests
from bs4 import BeautifulSoup
import os
# 爬取网页内容
url = 'https://www.example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
print(soup.prettify())
# 爬取图片
img_url = 'https://www.example.com/image.jpg'
response = requests.get(img_url)
img_name = os.path.basename(img_url)
with open(img_name, 'wb') as f:
f.write(response.content)
```
需要注意的是,爬取网页内容和图片时需要考虑网站的版权和法律问题,避免侵犯他人权益。同时,爬虫对网站的访问量也会产生一定压力,应该遵循网站的访问规则和礼仪。
python爬虫下载图片代码
好的,以下是一个简单的 Python 爬虫下载图片的代码示例:
```python
import requests
import os
# 如果不存在目标文件夹则创建
if not os.path.exists('images'):
os.makedirs('images')
url = 'https://www.example.com/image.jpg'
response = requests.get(url)
# 获取文件名
filename = url.split('/')[-1]
# 保存图片
with open('images/' + filename, 'wb') as f:
f.write(response.content)
print('已成功下载图片:' + filename)
```
您可以将 `url` 替换为目标图片的链接。在上述示例中,我们使用了 Python 的 requests 库来发送 HTTP 请求,然后将响应的内容保存到本地文件中。请注意,您需要确保您的代码具有下载目标图片的合法性。
阅读全文
相关推荐
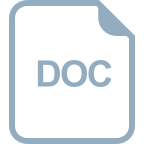
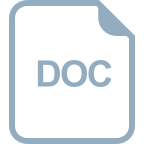
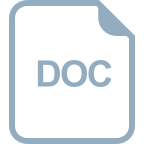
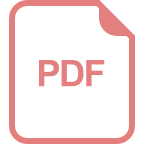
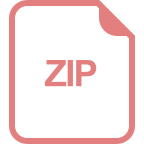
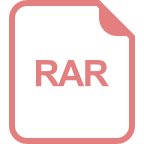
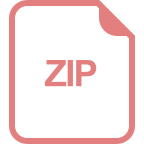



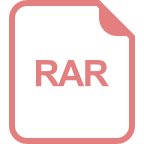
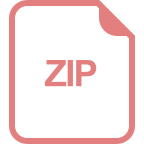
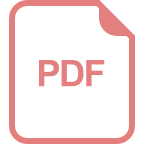
