链表的运用举三个列子用c语言
时间: 2023-06-03 22:03:21 浏览: 78
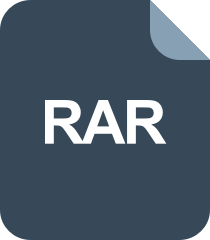
c语言实现链表的基本操作,链表是一种物理存储单元上非连续、非顺序的存储结构,数据元素的逻辑顺序是通过链表中的指针链接次序实现的。
好的,下面是三个链表的运用例子,使用C语言实现:
1. 链表实现简单计算器
#include <stdio.h>
#include <stdlib.h>
struct node {
char op;
float num;
struct node* next;
};
typedef struct node node;
int main() {
char ch;
float num, ans = 0;
node* head = NULL;
node* tail = NULL;
while (scanf("%c", &ch) != EOF) {
if (ch == '\n') {
break;
}
if (ch == '+' || ch == '-' || ch == '*' || ch == '/') {
node* n = malloc(sizeof(node));
n->op = ch;
n->next = NULL;
if (head == NULL) {
head = n;
tail = n;
} else {
tail->next = n;
tail = n;
}
} else {
ungetc(ch, stdin);
scanf("%f", &num);
node* n = malloc(sizeof(node));
n->num = num;
n->next = NULL;
if (head == NULL) {
head = n;
tail = n;
} else {
tail->next = n;
tail = n;
}
}
}
node* p = head;
while (p != NULL) {
if (p->op == '+') {
ans += p->next->num;
} else if (p->op == '-') {
ans -= p->next->num;
} else if (p->op == '*') {
ans *= p->next->num;
} else if (p->op == '/') {
ans /= p->next->num;
}
p = p->next;
}
printf("Ans: %.2f\n", ans);
return 0;
}
2. 链表实现队列
#include <stdio.h>
#include <stdlib.h>
struct node {
int data;
struct node* next;
};
typedef struct node node;
int main() {
node* head = NULL;
node* tail = NULL;
int n;
printf("Enter the number of elements to enqueue: ");
scanf("%d", &n);
for (int i = 0; i < n; i++) {
int x;
printf("Enter element %d: ", i + 1);
scanf("%d", &x);
node* n = malloc(sizeof(node));
n->data = x;
n->next = NULL;
if (head == NULL) {
head = n;
tail = n;
} else {
tail->next = n;
tail = n;
}
}
printf("Elements in the queue: ");
node* p = head;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
return 0;
}
3. 反转链表
#include <stdio.h>
#include <stdlib.h>
struct node {
int data;
struct node* next;
};
typedef struct node node;
int main() {
node* head = NULL;
int n;
printf("Enter the number of elements in the list: ");
scanf("%d", &n);
for (int i = 0; i < n; i++) {
int x;
printf("Enter element %d: ", i + 1);
scanf("%d", &x);
node* n = malloc(sizeof(node));
n->data = x;
n->next = head;
head = n;
}
printf("Original list: ");
node* p = head;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
node* prev = NULL;
node* curr = head;
node* next = NULL;
while (curr != NULL) {
next = curr->next;
curr->next = prev;
prev = curr;
curr = next;
}
printf("Reversed list: ");
p = prev;
while (p != NULL) {
printf("%d ", p->data);
p = p->next;
}
printf("\n");
return 0;
}
阅读全文
相关推荐
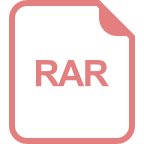
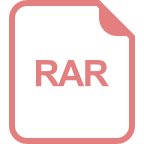
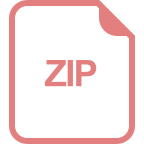
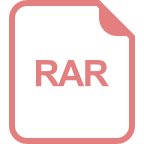
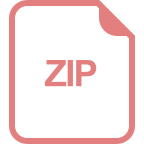
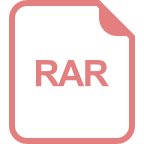
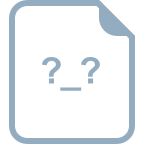
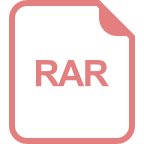
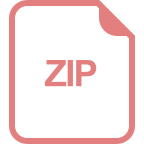
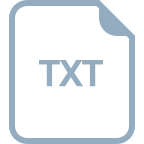