httpclient发送post请求案例
时间: 2023-08-13 20:06:49 浏览: 51
Sure! Here's an example of how to send a POST request using HttpClient in Java:
```java
import org.apache.http.HttpEntity;
import org.apache.http.HttpResponse;
import org.apache.http.client.HttpClient;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.HttpClientBuilder;
import org.apache.http.util.EntityUtils;
public class HttpClientExample {
public static void main(String[] args) {
HttpClient httpClient = HttpClientBuilder.create().build();
HttpPost httpPost = new HttpPost("http://www.example.com/api/endpoint");
try {
// Add request headers
httpPost.setHeader("Content-Type", "application/json");
// Add request body
String requestBody = "{\"key1\":\"value1\", \"key2\":\"value2\"}";
StringEntity stringEntity = new StringEntity(requestBody);
httpPost.setEntity(stringEntity);
// Send the POST request
HttpResponse response = httpClient.execute(httpPost);
// Get the response body
HttpEntity responseEntity = response.getEntity();
String responseBody = EntityUtils.toString(responseEntity);
// Print the response body
System.out.println(responseBody);
} catch (Exception e) {
e.printStackTrace();
}
}
}
```
In this example, we're using the Apache HttpClient library to send a POST request to `http://www.example.com/api/endpoint`. We set the request headers, add the request body as a JSON string, and then execute the request using `httpClient.execute(httpPost)`. Finally, we retrieve the response body from the `HttpResponse` object and print it out.
Remember to replace `http://www.example.com/api/endpoint` with the actual URL you want to send the POST request to, and modify the request headers and body according to your requirements.
相关推荐
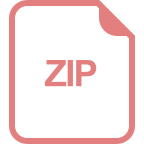
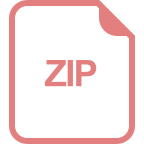
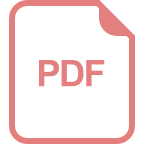














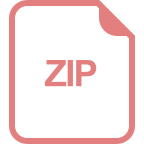