补全createThread函数,使用pthread_create函数创建线程,并将start_routine作为线程处理函数,arg作为线程处理函数的参数,同时将创建成功的线程ID作为createThread函数的返回值。
时间: 2023-08-11 10:25:27 浏览: 54
下面是补全后的代码:
```c
#include <pthread.h>
pthread_t createThread(void* (*start_routine)(void*), void* arg) {
pthread_t tid;
int ret = pthread_create(&tid, NULL, start_routine, arg);
if (ret != 0) {
// 创建线程失败
return -1;
}
return tid;
}
```
在上述代码中,我们使用pthread_create函数创建了一个线程,并将start_routine作为线程处理函数,arg作为线程处理函数的参数。如果创建线程成功,我们将创建成功的线程ID返回;如果创建线程失败,我们返回-1表示创建失败。
相关问题
createthread linux
在 Linux 中创建线程,可以使用 pthread 库提供的函数 pthread_create()。这个函数的原型如下:
```c
#include <pthread.h>
int pthread_create(pthread_t *thread, const pthread_attr_t *attr, void *(*start_routine) (void *), void *arg);
```
其中,参数 thread 是用来存放新创建的线程 ID 的指针;参数 attr 是用来设置新线程的属性的指针,一般可以传入 NULL;参数 start_routine 是新线程所要执行的函数的指针;参数 arg 是传递给新线程的函数的参数。
下面是一个简单的示例代码:
```c
#include <stdio.h>
#include <pthread.h>
void *thread_func(void *arg) {
printf("Hello from thread!\n");
return NULL;
}
int main() {
pthread_t tid;
pthread_create(&tid, NULL, thread_func, NULL);
pthread_join(tid, NULL);
return 0;
}
```
这个程序会创建一个新线程,并在新线程中执行 thread_func() 函数,该函数会输出一段信息。由于新线程是异步执行的,所以在主线程中需要调用 pthread_join() 函数等待新线程执行完毕。
线程的api是什么?api的参数是什么意思?类型是什么?如果参数是结构体,可能的结构体具体是什么?
线程的API是指操作系统或编程语言提供的用于创建、管理线程的一系列函数或方法。这些API允许程序员控制线程的生命周期,包括创建线程、结束线程、暂停线程、恢复线程等操作。
API的参数通常是指向特定数据的指针或数据本身,用于指定函数的操作细节。参数的类型可以是基本数据类型,也可以是指向结构体或类的指针。参数的具体含义取决于API的设计和它所执行的操作。
如果参数是结构体,那么这个结构体通常会包含一组用于描述线程属性的成员变量。这些结构体的具体内容会根据不同的操作系统和编程语言有所不同。例如,在Windows编程中,可以使用`SECURITY_ATTRIBUTES`结构体来指定新线程的属性,而在线程创建函数如`CreateThread`中,这个结构体可能作为参数之一,用于设置线程的安全属性。
一个典型的线程创建API的例子是POSIX线程(pthread)库中的`pthread_create`函数。其原型如下:
```c
int pthread_create(pthread_t *thread, const pthread_attr_t *attr, void *(*start_routine) (void *), void *arg);
```
参数解释:
- `pthread_t *thread`:指向线程标识符的指针,用于引用新创建的线程。
- `const pthread_attr_t *attr`:指向线程属性结构体的指针,该结构体定义了新线程的各种属性,如栈大小、调度策略等。如果没有特别需求,可以传递NULL来使用默认属性。
- `void *(*start_routine) (void *)`:指向线程开始执行函数的指针。线程创建后,会调用这个函数开始执行。
- `void *arg`:传递给`start_routine`函数的参数。
阅读全文
相关推荐
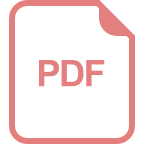
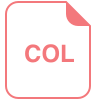
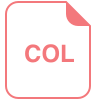
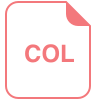
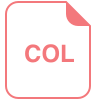

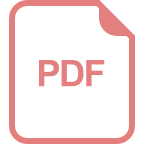
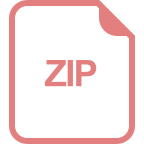
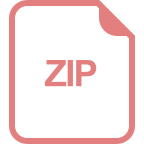
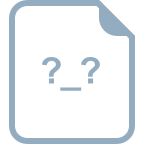
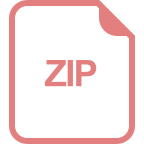
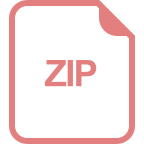
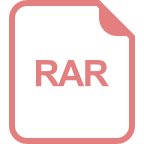